输出完整c++语言代码
时间: 2024-10-15 07:24:29 浏览: 17
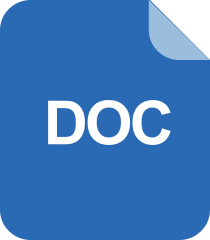
(完整版)C++经典程序代码大全.doc

在C++中,如果你需要创建一个函数来按照上述描述的方式拆分链表,你可以参考以下代码片段。这里假设`ListNode`是一个包含整数值并带有指针链接的链表结构:
```cpp
#include <iostream>
using namespace std;
// 链表节点定义
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {} // 构造函数
};
// 拆分链表函数
pair<ListNode*, ListNode*> splitList(ListNode* head) {
ListNode* L1_head = head; // L1链表的头节点
ListNode* L2_head = NULL; // L2链表的头节点(暂时无元素)
// 遍历链表
ListNode* current = head;
while (current != nullptr && current->next != nullptr) {
// 如果L2为空,或L2非空但仍有节点
if (L2_head == nullptr || L2_head->next != nullptr) {
// 添加当前节点到L2,并更新L1和L2的下一个节点
if (L2_head == nullptr) {
L2_head = current;
} else {
L1_next = L1_head->next;
L1_head->next = L2_head->next;
L2_head->next = L1_next;
}
// 移动到L1的下一个节点
L1_head = L1_head->next;
} else { // L2已满,不能再添加节点,直接跳出循环
break;
}
current = current->next;
}
return make_pair(L1_head, L2_head); // 返回L1和L2的头节点
}
// 测试函数
void printList(ListNode* head) {
ListNode* temp = head;
while (temp != nullptr) {
cout << temp->val << " ";
temp = temp->next;
}
cout << endl;
}
int main() {
// 创建示例链表
ListNode* listHead = new ListNode(1);
listHead->next = new ListNode(2);
listHead->next->next = new ListNode(3);
listHead->next->next->next = new ListNode(4);
pair<ListNode*, ListNode*> result = splitList(listHead);
cout << "Original List: ";
printList(listHead);
cout << "Split List: ";
printList(result.first);
cout << "Remaining List: ";
printList(result.second);
// 清理内存
delete listHead;
return 0;
}
```
这段代码首先定义了一个链表节点结构`ListNode`,接着编写了拆分链表的函数`splitList`,最后有测试函数`printList`用于查看结果。在`main`函数中,我们创建了一个简单的链表并对其进行拆分。
请注意,这只是一个基本的实现,实际应用中可能需要处理链表空的情况。同时,为了防止内存泄漏,在程序结束时别忘了删除动态分配的内存。
阅读全文
相关推荐
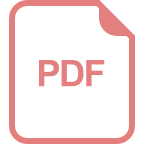
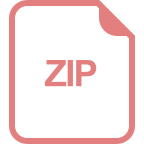















