双链表的基本操作c++新建,销毁,定位,查找,插入,删除,修改,输出的代码实现
时间: 2024-09-24 10:04:27 浏览: 39
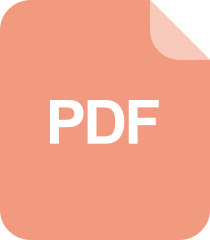
c++双向链表操作示例(创建双向链、双向链表中查找数据、插入数据等)
双链表是一种数据结构,其中每个节点包含两个指针,分别指向前一个节点和下一个节点。以下是C++中双链表的一些基本操作的代码实现:
1. **新建节点** (Node 类)
```cpp
class Node {
public:
int data;
Node* prev;
Node* next;
// 构造函数
Node(int value) : data(value), prev(nullptr), next(nullptr) {}
};
```
2. **新建双链表** (LinkedList 类)
```cpp
class LinkedList {
private:
Node* head;
Node* tail;
public:
LinkedList() : head(nullptr), tail(nullptr) {}
// 添加新节点到头部
void push_front(int value) {
Node* newNode = new Node(value);
if (!head) {
head = newNode;
tail = newNode;
} else {
newNode->next = head;
head->prev = newNode;
head = newNode;
}
}
// 等其他操作...
};
```
3. **销毁** (释放内存)
```cpp
// 删除头节点并调整链表
void delete_head(Node*& head) {
if (head) {
Node* temp = head;
head = head->next;
delete temp;
}
}
```
4. **定位、查找** (按值查找节点)
```cpp
Node* find_node(int value) const {
Node* current = head;
while (current && current->data != value) {
current = current->next;
}
return current;
}
// 查找后缀的第一个特定值
Node* find_first_value(int value) const {
Node* current = tail;
while (current && current->data != value) {
current = current->prev;
}
return current;
}
```
5. **插入、删除、修改** (假设`oldNode`是要被替换或移除的节点,`newValue`是新的值)
- 插入到头部:在`push_front`基础上添加
- 插入到尾部:找到尾节点,然后插入
- 删除:找到`oldNode`,更新相邻节点的指针
- 修改:找到`oldNode`,更改其`data`属性
6. **输出链表** (遍历打印)
```cpp
void print_list() const {
Node* current = head;
while (current) {
std::cout << current->data << " ";
current = current->next;
}
std::cout << std::endl;
}
```
阅读全文
相关推荐
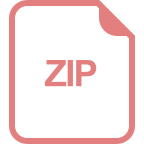
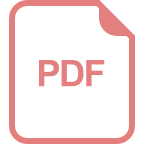
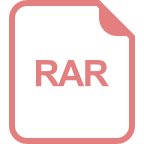
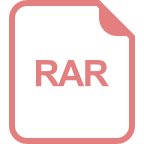
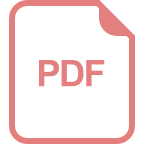
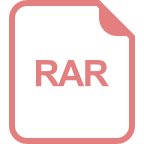
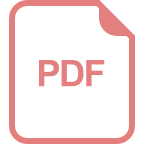
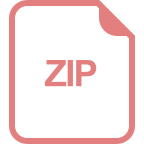
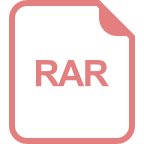
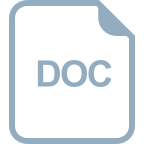
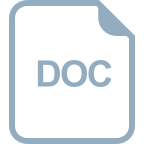
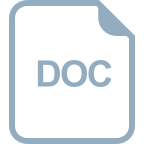
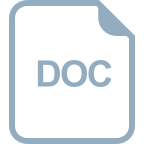
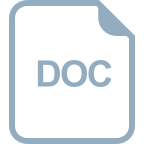
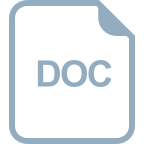