多元非线性回归模型 java代码
时间: 2023-08-20 08:02:27 浏览: 302
多元非线性回归模型是一种用于拟合多个自变量和一个因变量之间非线性关系的模型。在Java中,可以使用机器学习库或数值优化库来实现多元非线性回归模型的拟合。
以下是一个使用Apache Commons Math库实现多元非线性回归模型的示例代码:
```
import org.apache.commons.math3.fitting.leastsquares.LeastSquaresBuilder;
import org.apache.commons.math3.fitting.leastsquares.LeastSquaresProblem;
import org.apache.commons.math3.fitting.leastsquares.LevenbergMarquardtOptimizer;
import org.apache.commons.math3.fitting.leastsquares.MultivariateJacobianFunction;
import org.apache.commons.math3.fitting.leastsquares.ParameterGuesser;
import org.apache.commons.math3.linear.DiagonalMatrix;
import org.apache.commons.math3.util.FastMath;
import org.apache.commons.math3.util.Pair;
public class NonlinearRegression {
public static void main(String[] args) {
// 定义自变量和因变量
double[] variables = {1, 2, 3, 4, 5};
double[] observations = {2, 4, 6, 8, 10};
// 定义非线性模型函数
MultivariateJacobianFunction model = new MultivariateJacobianFunction() {
@Override
public Pair<double[], double[][]> value(double[] params) {
int numPoints = variables.length;
double[] values = new double[numPoints];
double[][] jacobian = new double[numPoints][params.length];
for (int i = 0; i < numPoints; i++) {
double x = variables[i];
double y = params[0] * FastMath.pow(x, params[1]); // 非线性模型函数
values[i] = y;
// 计算偏导数
jacobian[i][0] = FastMath.pow(x, params[1]);
jacobian[i][1] = params[0] * FastMath.pow(x, params[1]) * FastMath.log(x);
}
return new Pair<>(values, jacobian);
}
};
// 使用Levenberg-Marquardt优化算法拟合模型
double[] initialGuess = {1, 1}; // 初始参数猜测值
ParameterGuesser guesser = new ParameterGuesser(new LevenbergMarquardtOptimizer());
double[] bestFit = guesser.withModel(model).guess(variables, observations, initialGuess);
System.out.println("拟合参数:");
System.out.println("参数1:" + bestFit[0]);
System.out.println("参数2:" + bestFit[1]);
}
}
```
这段代码定义了一个含有两个参数的非线性模型函数,使用Levenberg-Marquardt优化算法拟合模型,并输出拟合得到的参数值。需要注意的是,实际应用中需要根据具体的非线性模型进行修改。
阅读全文
相关推荐
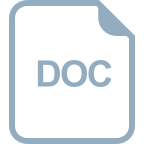
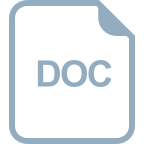
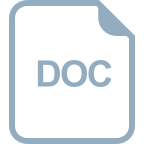
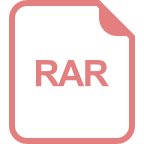
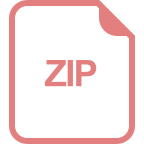
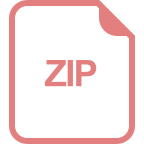
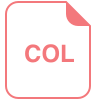
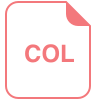
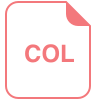
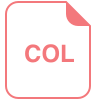
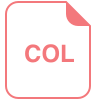
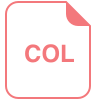
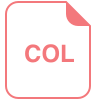
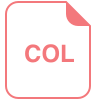
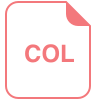
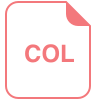
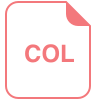
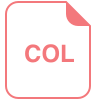
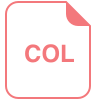