fgets和fputs文件的拷贝如何实现
时间: 2024-10-07 20:03:12 浏览: 51
在C语言中,如果需要从一个文件复制到另一个文件,可以使用标准库函数`fgets()`和`fputs()`。这两个函数分别用于从文件中读取一行文本和向文件写入一行文本。
以下是一个简单的示例,说明如何实现两个文件之间的内容拷贝:
```c
#include <stdio.h>
#include <string.h>
#define MAX_LINE_LENGTH 1024
int main() {
FILE *input_file, *output_file;
// 打开源文件和目标文件
if ((input_file = fopen("source.txt", "r")) == NULL) {
perror("Failed to open source file");
return 1;
}
if ((output_file = fopen("destination.txt", "w")) == NULL) {
perror("Failed to open destination file");
fclose(input_file);
return 1;
}
char line[MAX_LINE_LENGTH];
// 使用fgets逐行读取源文件的内容
while (fgets(line, sizeof(line), input_file)) {
// 使用fputs将内容写入目标文件
fputs(line, output_file);
}
// 处理末尾换行符
if (!feof(input_file)) {
fseek(input_file, -1, SEEK_CUR); // 移动到末尾减去1(跳过换行)
line[strlen(line) - 1] = '\0'; // 删除末尾的换行符
fputs(line, output_file);
}
// 关闭文件
fclose(input_file);
fclose(output_file);
printf("Copy successful.\n");
return 0;
}
```
这个程序首先打开两个文件,然后使用`fgets`逐行读取源文件的内容,并使用`fputs`写入目标文件。最后别忘了关闭文件处理可能出现的错误。
阅读全文
相关推荐
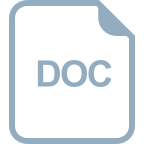
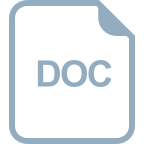
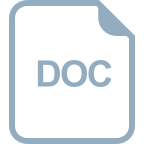
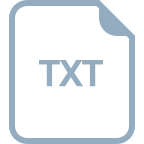
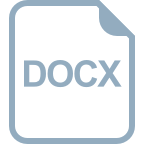
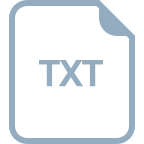
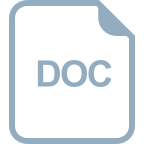
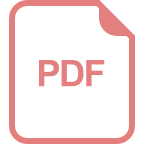
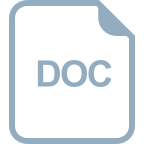
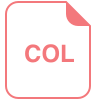
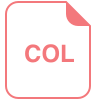
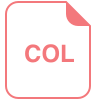
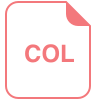
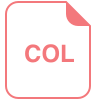
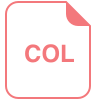
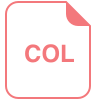
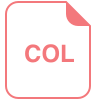

