输入一个字符串,以回车结束(字符串长度不超过100)。 该字符串由若干个单词组成,单词之间用一个空格隔开,所有单词区分大写。现需要将其中的某个单词替换成另一个单词,并输出替换之后的字符串。
时间: 2024-12-18 10:30:34 浏览: 9
你可以通过使用 C++ 中的 `std::string` 和 `substr()` 函数来实现这个功能。下面是一个简单的函数示例,它接受两个参数:原始字符串和需要替换的旧词以及新词:
```cpp
#include <iostream>
#include <string>
std::string replaceWord(std::string input, const std::string& old_word, const std::string& new_word) {
size_t pos = input.find(old_word); // 查找旧词的位置
while (pos != std::string::npos) {
// 替换旧词
input.replace(pos, old_word.length(), new_word);
// 继续查找下一个旧词
pos = input.find(old_word, pos + new_word.length());
}
return input;
}
int main() {
std::string str, old_word, new_word;
std::cout << "Enter a string (max 100 characters): ";
getline(std::cin, str);
std::cout << "Enter the word to be replaced: ";
getline(std::cin, old_word);
std::cout << "Enter the replacement word: ";
getline(std::cin, new_word);
std::string result = replaceWord(str, old_word, new_word);
std::cout << "Replaced string: " << result << "\n";
return 0;
}
```
这个程序首先读取用户输入的字符串、旧词和新词,然后在原始字符串中查找旧词并替换之,直到找不到更多旧词为止。
阅读全文
相关推荐
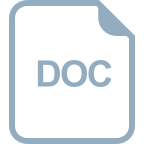
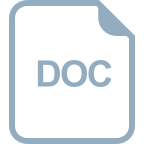
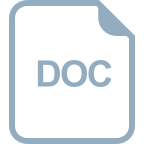






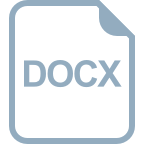
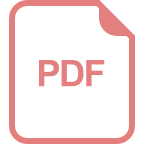
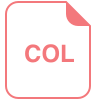
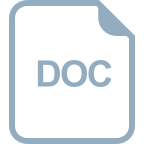
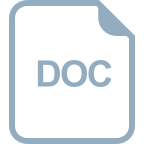
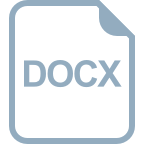