编写一个函数,由实参传来一个字符串,统计此字符串中字母、数字、空格和其他字符的个数,在主函数中输入字符串以及输出上述的结果。用C语言,不用指针和不常见函数
时间: 2024-10-20 19:02:34 浏览: 28
当然可以。下面是一个简单的C程序,它使用基本的控制结构来完成这个任务,没有使用指针或不常见的库函数:
```c
#include <stdio.h>
#include <ctype.h> // 引入ctype.h以获取字符分类函数
void count_chars(char str[]) {
int alpha_count = 0; // 字母计数
int digit_count = 0; // 数字计数
int space_count = 0; // 空格计数
int other_count = 0; // 其他字符计数
for (int i = 0; str[i] != '\0'; i++) { // 遍历字符串直到结束
if (isalpha(str[i])) { // 判断是否为字母
alpha_count++;
} else if (isdigit(str[i])) { // 判断是否为数字
digit_count++;
} else if (str[i] == ' ') { // 如果是空格
space_count++;
} else { // 对于其他字符(不是字母、数字或空格)
other_count++;
}
}
printf("字母: %d\n", alpha_count);
printf("数字: %d\n", digit_count);
printf("空格: %d\n", space_count);
printf("其他字符: %d\n", other_count);
}
int main() {
char input[100]; // 输入字符串的数组
printf("请输入一个字符串: ");
fgets(input, sizeof(input), stdin); // 从标准输入读取字符串,包括换行符
// 去除fgets读取到的换行符
input[strlen(input) - 1] = '\0';
count_chars(input);
return 0;
}
```
在这个程序中,`count_chars` 函数遍历输入的字符串,并使用 `isalpha` 和 `isdigit` 来判断字符类型。然后,`main` 函数接收用户输入,调用 `count_chars` 并打印结果。
阅读全文
相关推荐
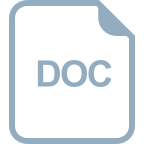
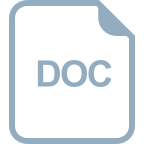
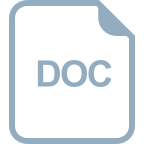















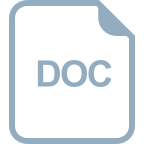