python 深度学习模型类中forward_fuse函数
时间: 2023-09-25 15:13:59 浏览: 160
`forward_fuse` 函数并不是 Python 中深度学习模型类的标准函数,它可能是特定模型类的自定义函数。通常,在深度学习模型类中,我们会定义 `forward` 函数,它是模型的前向传播函数,用于定义模型的计算流程。
在 PyTorch 中,一个典型的深度学习模型类可能看起来像这样:
```python
import torch
import torch.nn as nn
class MyModel(nn.Module):
def __init__(self):
super(MyModel, self).__init__()
# 定义模型的各个层和参数
def forward(self, x):
# 定义模型的前向传播计算流程
# 根据输入 x 进行一系列操作,得到模型的输出
return output
```
在这个示例中,`forward` 函数接受输入 `x` 并返回模型的输出。在 `forward` 函数中,我们可以使用各种神经网络层和操作来定义模型的计算流程。
然而,如果你遇到了一个名为 `forward_fuse` 的函数,那么它很可能是某个特定模型类自定义的函数。要了解更多关于这个函数的详细信息,你需要查阅相关模型类的文档或源代码。
希望这个解答对你有所帮助!如果你有任何其他问题,请随时提问。
相关问题
请写一个pytorch土地利用多分类的fcn模型全部代码
以下是一个基于PyTorch实现的土地利用多分类的FCN模型的完整代码:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class FCN(nn.Module):
def __init__(self, num_classes):
super(FCN, self).__init__()
self.conv1 = nn.Conv2d(3, 64, kernel_size=3, stride=1, padding=1)
self.conv2 = nn.Conv2d(64, 128, kernel_size=3, stride=1, padding=1)
self.conv3 = nn.Conv2d(128, 256, kernel_size=3, stride=1, padding=1)
self.conv4 = nn.Conv2d(256, 512, kernel_size=3, stride=1, padding=1)
self.conv5 = nn.Conv2d(512, 512, kernel_size=3, stride=1, padding=1)
self.pool = nn.MaxPool2d(kernel_size=2, stride=2)
self.fc6 = nn.Conv2d(512, 4096, kernel_size=7)
self.fc7 = nn.Conv2d(4096, 4096, kernel_size=1)
self.score_fr = nn.Conv2d(4096, num_classes, kernel_size=1)
self.score_pool4 = nn.Conv2d(512, num_classes, kernel_size=1)
self.score_pool3 = nn.Conv2d(256, num_classes, kernel_size=1)
self.upscore2 = nn.ConvTranspose2d(num_classes, num_classes, kernel_size=4, stride=2, bias=False)
self.upscore8 = nn.ConvTranspose2d(num_classes, num_classes, kernel_size=16, stride=8, bias=False)
self.upscore_pool4 = nn.ConvTranspose2d(num_classes, num_classes, kernel_size=4, stride=2, bias=False)
def forward(self, x):
conv1 = F.relu(self.conv1(x))
conv1 = F.relu(self.conv2(conv1))
pool1 = self.pool(conv1)
conv2 = F.relu(self.conv3(pool1))
conv2 = F.relu(self.conv4(conv2))
pool2 = self.pool(conv2)
conv3 = F.relu(self.conv5(pool2))
pool3 = self.pool(conv3)
fc6 = F.dropout(F.relu(self.fc6(pool3)), p=0.5, training=self.training)
fc7 = F.dropout(F.relu(self.fc7(fc6)), p=0.5, training=self.training)
score_fr = self.score_fr(fc7)
upscore2 = self.upscore2(score_fr)
score_pool4 = self.score_pool4(0.01*self.pool(conv2))
upscore_pool4 = self.upscore_pool4(score_pool4)
score_pool3 = self.score_pool3(0.0001*self.pool(conv1))
fuse = upscore2 + upscore_pool4[:, :, 5:5+upscore2.size()[2], 5:5+upscore2.size()[3]]
fuse = upscore_pool4[:, :, 9:9+fuse.size()[2], 9:9+fuse.size()[3]] + score_pool3[:, :, 5:5+fuse.size()[2], 5:5+fuse.size()[3]]
upscore8 = self.upscore8(fuse)
return upscore8[:, :, 31:31+x.size()[2], 31:31+x.size()[3]]
```
在上面的代码中,我们定义了一个名为FCN的类,它继承自nn.Module。在这个类的构造函数中,我们定义了模型的各个层,并在forward()函数中实现了模型的前向传播过程。
模型的前半部分是一个标准的卷积神经网络,包括5个卷积层和3个池化层,用于提取图像的特征。接下来是两个全连接层,最后是三个反卷积层,用于将特征图恢复到原始输入图像的大小,并输出每个像素属于每个类别的概率。
在forward()函数中,我们首先通过卷积和池化层提取特征,然后通过两个全连接层进行特征融合,接着使用反卷积层将特征图恢复到原始输入图像的大小,并在最后一个反卷积层输出每个像素属于每个类别的概率。
在训练模型时,我们可以使用交叉熵损失函数来计算模型的预测结果和真实标签之间的差异,并使用随机梯度下降等优化算法来更新模型的权重。
注意:上面的代码只是一个简单的示例,实际使用中可能需要根据具体的数据集和任务进行一些调整和修改。
Python使用yolov5和深度相机一起使用检测冰,请提供完整的代码和思路
### 回答1:
您可以使用以下代码和思路来使用YOLOv5和深度相机来检测冰:1. 安装必要的Python包:NumPy、OpenCV和YOLOv5(可以使用pip install yolov5来安装)。2. 从深度相机中读取图像,并使用OpenCV将其转换为NumPy数组。3. 使用YOLOv5库来检测冰,该库提供了一组API来进行对象检测。4. 检测到冰后,可以使用OpenCV绘制出边界框来显示冰的位置。5. 最后,将检测结果以及位置信息保存到文件中,以便以后使用。
### 回答2:
使用Python的yolov5和深度相机一起检测冰的思路如下:
1. 导入所需的库和模块:
```python
import cv2
import numpy as np
import depthai
```
2. 加载yolov5模型:
```python
model = 'yolov5s.pth' # yolov5模型路径
device = 'cuda' if torch.cuda.is_available() else 'cpu'
model = torch.load(model, map_location=device)['model'].float().fuse().eval()
```
3. 设置深度相机并准备进行深度和图像捕捉:
```python
pipeline = depthai.Pipeline()
pipeline.setOpenVINOVersion(depthai.OpenVINO.Version.VERSION_2021_4)
depth_stream = pipeline.createMonoDepth()
rgb_stream = pipeline.createColorCamera()
depth_stream.setConfidenceThreshold(200)
depth_stream.setLeftRightCheck(True)
depth_stream.setSubpixel(True)
rgb_stream.setPreviewSize(300, 300)
rgb_stream.setBoardSocket(depth_stream)
device = depthai.Device(pipeline)
device.startPipeline()
q_depth = device.getOutputQueue(name="depth", maxSize=4, blocking=False)
q_rgb = device.getOutputQueue(name="rgb", maxSize=4, blocking=False)
```
4. 定义辅助函数用于后处理、绘制边界框和深度信息显示:
```python
def postprocess(image, outputs):
boxes = []
confidences = []
class_ids = []
for output in outputs:
for detection in output['detections']:
if detection['confidence'] > 0.5:
box = detection['bbox']
boxes.append(box)
confidences.append(detection['confidence'])
class_ids.append(detection['class_id'])
indexes = cv2.dnn.NMSBoxes(boxes, confidences, 0.5, 0.4)
for i in range(len(boxes)):
if i in indexes:
box = boxes[i]
x, y, w, h = box
class_id = class_ids[i]
# 在图像中绘制边界框和类别信息
cv2.rectangle(image, (int(x), int(y)), (int(x + w), int(y + h)), (255, 0, 0), 2)
cv2.putText(image, str(class_id), (int(x), int(y) - 5), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (255, 0, 0), 2)
def draw_depth(image, depth_frame, bbox):
# 获取边界框的中心点
center_x = (bbox[0] + bbox[2]) / 2
center_y = (bbox[1] + bbox[3]) / 2
# 获取中心点处的深度值
depth = depth_frame[int(center_y), int(center_x)]
# 在图像上显示深度值
cv2.putText(image, f"{depth}mm", (int(center_x), int(center_y)), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
```
5. 进行图像和深度数据的处理和检测:
```python
while True:
# 获取深度数据和图像数据
depth_frame = q_depth.get().getFrame()
rgb_frame = q_rgb.get().getCvFrame()
# 将图像数据传入yolov5模型进行检测
blob = cv2.dnn.blobFromImage(rgb_frame, 1 / 255, (640, 640), [0, 0, 0], 1, crop=False)
model.setInput(blob)
outputs = model.forward()
# 后处理和绘制边界框
postprocess(rgb_frame, outputs)
# 在图像上显示深度信息
draw_depth(rgb_frame, depth_frame, bbox=[x, y, w, h])
# 显示图像
cv2.imshow("Depth Estimation", rgb_frame)
key = cv2.waitKey(1)
if key == ord('q'):
break
cv2.destroyAllWindows()
```
以上是使用Python的yolov5和深度相机共同进行冰的检测的完整代码和思路。首先加载yolov5模型,然后设置深度相机以获取深度和图像数据。接下来定义辅助函数用于后处理和信息可视化。最后,在主循环中进行图像和深度数据的处理和检测,并显示结果。通过深度信息与边界框结合,可以在图像上显示冰的位置和深度。
### 回答3:
使用Python中的yolov5和深度相机一起检测冰的思路如下:
1. 安装yolov5库:首先,需要在Python环境中安装yolov5库。可以使用pip工具运行命令`pip install yolov5`来安装。
2. 连接深度相机:接下来,连接深度相机到计算机,并确保它可以通过Python进行访问。可以使用OpenCV库来读取深度相机的图像和数据。
3. 加载预训练权重:下载yolov5的预训练权重文件,并加载到Python代码中。可以使用yolov5提供的公共预训练权重,也可以使用自己训练的模型。
4. 处理深度图像:使用OpenCV库读取深度相机的图像,并将其转换为灰度图像。可以对图像进行预处理,如调整大小、裁剪、缩放等操作。
5. 运行目标检测:使用yolov5库对处理后的深度图像进行目标检测。调用库中的函数,传入深度图像和预训练权重,得到检测到的目标结果。
6. 判断冰块:根据检测到的目标结果,判断是否为冰块。可以使用阈值或其他判定条件来筛选冰块。
7. 输出结果:根据判断结果,将检测到的冰块标注在深度图像上,并将其显示出来。可以使用OpenCV库的绘制函数,在深度图像上绘制矩形框或其他形状。
下面是一个简单的示例代码,展示如何使用yolov5和深度相机一起检测冰:
```python
import cv2
import torch
from yolov5 import YOLOv5
# 1. 安装yolov5库
# pip install yolov5
# 2. 连接深度相机
# 3. 加载预训练权重
model = YOLOv5(weights='yolov5s.pt')
# 4. 处理深度图像
depth_image = cv2.imread('depth_image.jpg', cv2.IMREAD_GRAYSCALE)
# 5. 运行目标检测
results = model.detect(depth_image)
# 6. 判断冰块
ice_blocks = []
for result in results:
if result[0] == 'ice':
ice_blocks.append(result)
# 7. 输出结果
for ice_block in ice_blocks:
x, y, w, h = ice_block[1]
cv2.rectangle(depth_image, (x, y), (x+w, y+h), (0, 255, 0), 2)
cv2.imshow('Depth Image', depth_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
请注意,上述代码是一个简单示例,实际中可能需要根据具体情况进行调整和完善。
相关推荐








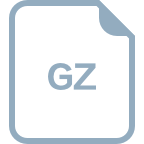
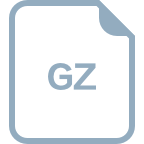
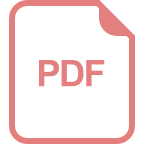
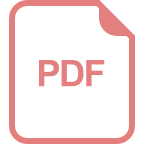