c++ 去掉一个长字符串得换行符,重新拼接为一个长字符串
时间: 2024-09-06 10:06:37 浏览: 36
在 C++ 中,如果你想去掉一个长字符串中的所有换行符并重新拼接成一个字符串,你可以使用 `std::string` 的 `erase` 和 `replace` 函数。假设 `str` 是你要处理的字符串,可以按照下面的步骤操作:
1. 使用 `find_last_of` 查找最后一个换行符的位置,它会返回第一个找到的字符的位置,如果找不到则返回字符串长度。
```cpp
size_t newline_pos = str.find_last_of("\n");
```
2. 如果找到了换行符,则从那个位置开始到字符串结束删除所有的换行符。这里使用 `erase` 函数从 `newline_pos+1` 开始到字符串结尾。
```cpp
if (newline_pos != std::string::npos) {
str.erase(newline_pos + 1, str.length());
}
```
3. 如果你想添加一个新的换行符,可以在适当的位置插入一个 `\n` 字符。例如,在原字符串最后添加一个换行符:
```cpp
if (newline_pos == std::string::npos) {
str += "\n";
} else {
str.insert(str.begin() + newline_pos, '\n');
}
```
4. 最后得到的就是去掉了换行符并可能新增了换行的新字符串。
完整的示例:
```cpp
#include <iostream>
#include <string>
std::string remove_newlines(const std::string& input_str) {
size_t newline_pos = input_str.find_last_of("\n");
if (newline_pos != std::string::npos) {
input_str.erase(newline_pos + 1, input_str.length());
}
if (newline_pos == stdstring::npos) {
input_str += "\n";
} else {
input_str.insert(input_str.begin() + newline_pos, '\n');
}
return input_str;
}
int main() {
std::string str = "这是一个包含\n换行符的长字符串.";
std::cout << remove_newlines(str) << std::endl;
return 0;
}
```
相关推荐
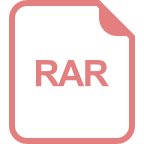
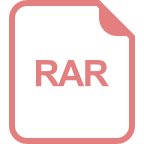
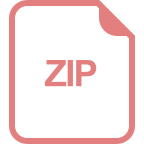



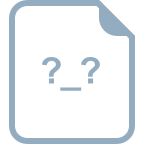
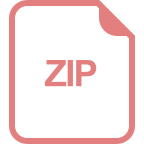
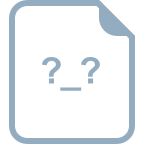
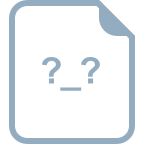
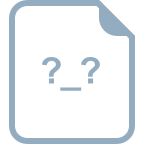
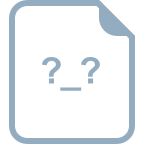