java 实现 redis 数据转成mysql存储
时间: 2024-04-15 09:31:12 浏览: 232
要将 Redis 中的数据转存到 MySQL 中,你可以使用 Java 编写一个程序来实现。下面是一个简单的示例代码,供你参考:
```java
import redis.clients.jedis.Jedis;
import redis.clients.jedis.ScanParams;
import redis.clients.jedis.ScanResult;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.Map;
public class RedisToMysql {
public static void main(String[] args) {
// Redis 连接参数
String redisHost = "localhost";
int redisPort = 6379;
String redisPassword = "your_redis_password";
int redisDbIndex = 0;
// MySQL 连接参数
String mysqlHost = "localhost";
int mysqlPort = 3306;
String mysqlDatabase = "your_database_name";
String mysqlUsername = "your_mysql_username";
String mysqlPassword = "your_mysql_password";
// 连接 Redis
Jedis jedis = new Jedis(redisHost, redisPort);
jedis.auth(redisPassword);
jedis.select(redisDbIndex);
// 连接 MySQL
try (Connection conn = DriverManager.getConnection("jdbc:mysql://" + mysqlHost + ":" + mysqlPort + "/" + mysqlDatabase, mysqlUsername, mysqlPassword)) {
// 遍历 Redis 中的所有键
ScanParams scanParams = new ScanParams().match("*");
ScanResult<String> scanResult;
String cursor = "0";
do {
scanResult = jedis.scan(cursor, scanParams);
for (String key : scanResult.getResult()) {
// 从 Redis 获取数据
Map<String, String> redisData = jedis.hgetAll(key);
// 将数据插入到 MySQL
String sql = "INSERT INTO your_table_name (key, field, value) VALUES (?, ?, ?)";
try (PreparedStatement stmt = conn.prepareStatement(sql)) {
for (Map.Entry<String, String> entry : redisData.entrySet()) {
stmt.setString(1, key);
stmt.setString(2, entry.getKey());
stmt.setString(3, entry.getValue());
stmt.executeUpdate();
}
}
}
cursor = scanResult.getStringCursor();
} while (!cursor.equals("0"));
System.out.println("Data transfer completed!");
} catch (SQLException e) {
e.printStackTrace();
} finally {
jedis.close();
}
}
}
```
请根据你的实际情况修改示例代码中的 Redis 和 MySQL 连接参数,以及 MySQL 表名等信息。这段代码将遍历 Redis 中的所有键,将键值对数据插入到 MySQL 的指定表中。你还可以根据实际需求进行进一步的优化和扩展。
阅读全文
相关推荐
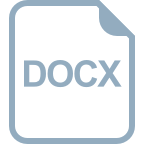
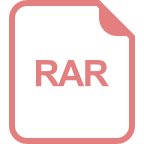

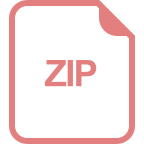
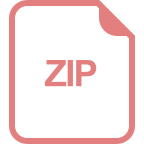
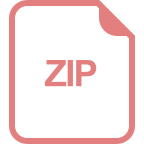
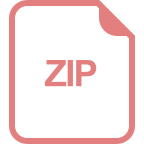
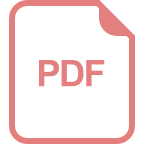
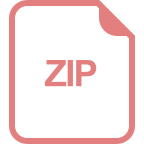
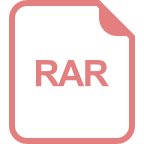
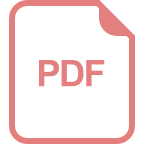
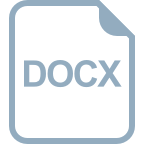
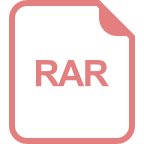
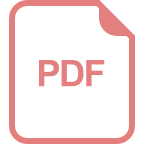
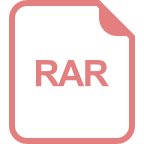
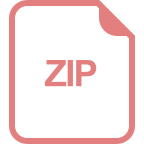