通过C++完成数字信封
时间: 2024-12-18 19:24:10 浏览: 6
在C++中,数字信封(Digital Envelope)通常是一个用于保护信息传输安全的概念,它将消息(明文)加密并与接收者的公钥一起发送。这个过程涉及两个步骤:封装(enveloping)和解封装(unenveloping)。以下是一个简单的概念实现:
1. **生成并加载公钥私钥对**:
使用库如OpenSSL,可以创建一个RSA密钥对,公开的公钥用于加密,私钥用于解密。
```cpp
#include <openssl/pem.h>
#include <openssl/rsa.h>
RSA* gen_rsa_key() {
RSA *rsa = RSA_new();
// ...生成并初始化RSA key...
return rsa;
}
```
2. **封装(加密)**:
使用接收者的公钥,对明文数据进行RSA加密。
```cpp
std::string encrypt(const std::string& plaintext, const RSA* recipient_pubkey) {
int len;
unsigned char ciphertext[PKCS1_OAEP_MAX_SSL3];
BIGNUM *bn = NULL;
if (!RSA_public_encrypt(plaintext.length(), (const unsigned char*)plaintext.c_str(), ciphertext, recipient_pubkey, PKCS1_OAEP_PADDING, &len)) {
// 错误处理...
}
BN_free(bn);
return std::string((char*)ciphertext, len);
}
```
3. **解封装(解密)**:
接收者收到加密的数据后,使用其私钥进行解密。
```cpp
std::string decrypt(const std::string& ciphertext, RSA* sender_privkey) {
int len;
unsigned char decrypted_text[1024]; // 假设足够大存储解密后的文本
BIGNUM *bn = NULL;
if (!RSA_private_decrypt(ciphertext.size(), (unsigned char*)ciphertext.c_str(), decrypted_text, sender_privkey, PKCS1_OAEP_PADDING, &len)) {
// 错误处理...
}
BN_free(bn);
return std::string(decrypted_text, len);
}
```
阅读全文
相关推荐







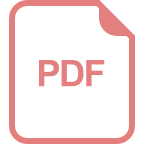




