python脚本调用git bash 输入命令 并得到返回结果
时间: 2024-09-18 13:14:54 浏览: 55
在Python脚本中调用Git Bash(Git的命令行界面)并获取其命令的结果,你可以使用os模块的`subprocess`函数。这个函数允许你在Python程序中运行外部命令,并接收其输出。下面是一个简单的例子:
```python
import subprocess
def run_git_command(command):
# 创建一个子进程来执行git命令
git_process = subprocess.Popen(['git', command], stdout=subprocess.PIPE, stderr=subprocess.PIPE)
# 等待命令完成并获取输出
output, error = git_process.communicate()
# 将输出解码为字符串(如果是二进制)
output_str = output.decode('utf-8')
# 检查是否有错误
if error:
print(f"Error: {error.decode('utf-8')}")
return output_str
# 调用示例:获取当前仓库的版本号
version = run_git_command("rev-parse HEAD")
print(f"Version: {version}")
#
相关问题
python创建git仓库
在Python中,虽然Git本身不是通过语言内置的功能来管理的,但是你可以使用Python脚本来辅助Git操作。首先,你需要确保已经在本地安装了Git。如果你想在项目目录下创建一个新的Git仓库,可以按照以下步骤操作:
1. **打开终端或命令提示符** (如果你是在Windows上,建议使用Git Bash或PowerShell)。
2. **导航到项目文件夹**,例如:
```
cd /path/to/your/project
```
3. **初始化新的Git仓库**,运行:
```
git init
```
这将创建一个名为`.git`的隐藏目录,存储所有Git相关的元数据。
4. **添加文件至暂存区**,然后提交更改:
- 添加所有文件:
```
git add .
```
- 提交更改并输入提交信息:
```
git commit -m "Initial commit"
```
5. **关联远程仓库(如果需要)**:
- 首先克隆一个已存在的GitHub仓库:
```
git clone https://github.com/username/repository.git
```
- 或者连接到一个现有的远程仓库:
```
git remote add origin https://github.com/username/repository.git
git push -u origin main
```
6. **跟踪分支和合并**,使用`git branch`, `git checkout`, 和 `git merge`等命令进行日常管理。
注意,这只是基本的操作流程,实际使用过程中可能会有更多高级选项和配置。如果你需要编写自动化脚本,可以考虑使用`subprocess`模块调用Git的命令行工具。
阅读全文
相关推荐
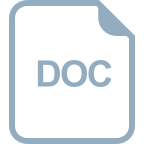
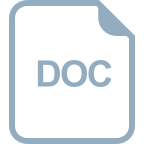
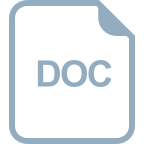
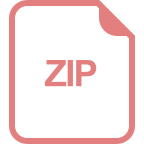
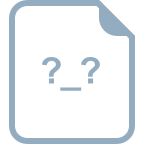
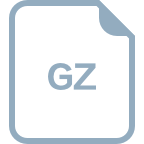
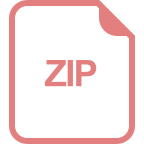
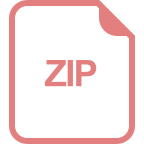
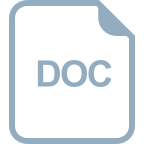
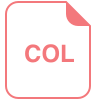
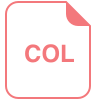
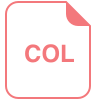
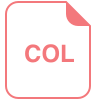
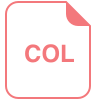
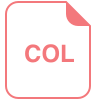
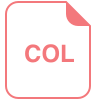
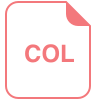
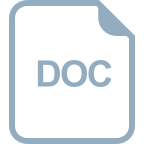