Java获取excel数据
时间: 2024-10-08 16:15:19 浏览: 22
在Java中,获取Excel数据通常需要使用第三方库,如Apache POI、JExcelApi或者是最近比较流行的_excel4j等。以下是使用Apache POI的一个简单示例:
```java
import org.apache.poi.ss.usermodel.*;
import java.io.File;
public class ExcelReader {
public static void main(String[] args) {
try {
FileInputStream fis = new FileInputStream(new File("path_to_your_file.xlsx")); // 替换为你的Excel文件路径
Workbook workbook = WorkbookFactory.create(fis);
Sheet sheet = workbook.getSheetAt(0); // 获取第一个工作表
Iterator<Row> rowIterator = sheet.iterator();
while (rowIterator.hasNext()) {
Row currentRow = rowIterator.next();
for (Cell cell : currentRow) {
switch (cell.getCellType()) {
case STRING:
System.out.print(cell.getStringCellValue() + "\t");
break;
case NUMERIC:
System.out.print(cell.getNumericCellValue() + "\t");
break;
case BOOLEAN:
System.out.print(cell.getBooleanCellValue() + "\t");
break;
default:
System.out.print(cell.toString() + "\t");
}
}
System.out.println(); // 每行数据打印完后换行
}
workbook.close();
fis.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
在这个例子中,我们首先创建了一个`Workbook`对象,然后读取第一个工作表,遍历每一行并处理每个单元格的数据。你需要根据实际需求调整代码以适应你的Excel文件结构。
阅读全文
相关推荐
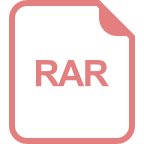
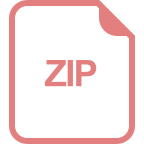
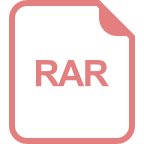
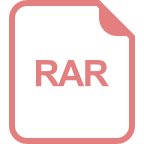
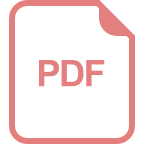
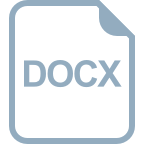
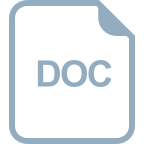
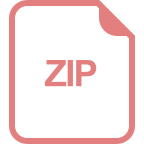
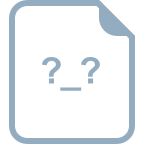
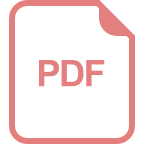
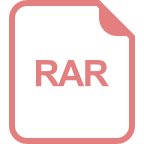
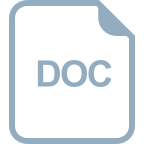
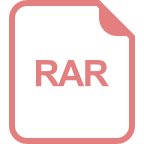
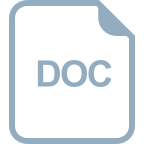
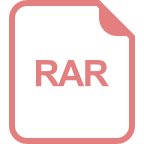
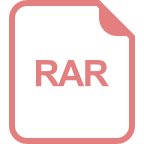