在Unity3D中如何使用C#脚本通过`transform.Rotate`, `OnMouseDown`, `OnMouseDrag`方法实现球体的鼠标拖拽旋转和移动?
时间: 2024-11-10 18:20:22 浏览: 9
为了实现鼠标拖拽旋转和移动球体的功能,我们需要编写C#脚本来捕捉和处理Unity中的鼠标事件。以下是实现这一功能的详细步骤和代码示例:
参考资源链接:[Unity3D C# 实现鼠标拖拽旋转与移动球体](https://wenku.csdn.net/doc/47tz14113q?spm=1055.2569.3001.10343)
首先,创建一个C#脚本命名为`DraggableSphere`,并将其附加到你想要拖拽的球体对象上。
接下来,在脚本中定义必要的变量来存储旋转和移动相关的数据:
```csharp
public class DraggableSphere : MonoBehaviour
{
private Vector3 m_RotationAxis = Vector3.zero;
private Vector3 m_LastMousePosition = Vector3.zero;
private float m_DragSpeed = 0.0f;
private bool m_IsDragging = false;
}
```
然后,实现`OnMouseDown`方法来开始拖拽过程:
```csharp
void OnMouseDown()
{
m_LastMousePosition = Input.mousePosition;
m_IsDragging = true;
}
```
之后,实现`OnMouseDrag`方法来处理鼠标拖拽过程中的旋转和移动:
```csharp
void OnMouseDrag()
{
Vector3 currentMousePosition = Input.mousePosition;
Vector3 delta = currentMousePosition - m_LastMousePosition;
// 计算旋转轴,这里可以根据需要调整
m_RotationAxis.x = -delta.y;
m_RotationAxis.y = delta.x;
// 根据旋转轴和鼠标移动速度计算旋转角度
transform.Rotate(m_RotationAxis, Space.World);
// 更新上一次鼠标位置
m_LastMousePosition = currentMousePosition;
// 记录拖拽状态和速度
m_IsDragging = true;
}
```
最后,编写`Update`方法来处理拖拽停止后的逻辑:
```csharp
void Update()
{
// 如果鼠标释放,则逐渐减小旋转速度,模拟惯性
if (!m_IsDragging)
{
if (m_DragSpeed > 0)
{
m_DragSpeed -= Time.deltaTime;
m_DragSpeed = Mathf.Max(0, m_DragSpeed);
}
else
{
m_IsDragging = false;
}
}
}
```
通过以上步骤,我们可以在Unity3D中使用C#脚本实现鼠标拖拽来控制球体的旋转和移动。建议详细阅读《Unity3D C# 实现鼠标拖拽旋转与移动球体》教程,进一步了解`DragRotateWithSlider`和`DragRotateLevel`脚本的具体实现和应用场景。
参考资源链接:[Unity3D C# 实现鼠标拖拽旋转与移动球体](https://wenku.csdn.net/doc/47tz14113q?spm=1055.2569.3001.10343)
阅读全文
相关推荐
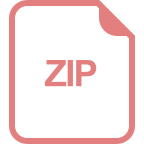
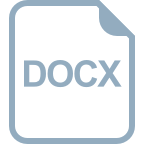
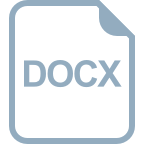















