java 双线性插值 代码
时间: 2023-08-08 18:01:50 浏览: 95
双线性插值是一种用于图像处理中的插值方法,常用于图像的放大、缩小等操作。在Java中,我们可以使用以下代码实现双线性插值。
```java
import java.awt.*;
import java.awt.image.*;
public class BilinearInterpolation {
public static BufferedImage bilinearInterpolation(BufferedImage image, int newWidth, int newHeight) {
int oldWidth = image.getWidth();
int oldHeight = image.getHeight();
BufferedImage newImage = new BufferedImage(newWidth, newHeight, image.getType());
Graphics2D g2d = newImage.createGraphics();
for (int i = 0; i < newWidth; i++) {
for (int j = 0; j < newHeight; j++) {
int x = (int) ((double) i / newWidth * oldWidth);
int y = (int) ((double) j / newHeight * oldHeight);
int x1 = Math.min(x + 1, oldWidth - 1);
int y1 = Math.min(y + 1, oldHeight - 1);
int rgb00 = image.getRGB(x, y);
int rgb01 = image.getRGB(x, y1);
int rgb10 = image.getRGB(x1, y);
int rgb11 = image.getRGB(x1, y1);
int interpolatedRGB = bilinearInterpolate(rgb00, rgb01, rgb10, rgb11, x, y, x1, y1, newWidth, newHeight);
newImage.setRGB(i, j, interpolatedRGB);
}
}
g2d.dispose();
return newImage;
}
private static int bilinearInterpolate(int rgb00, int rgb01, int rgb10, int rgb11,
int x, int y, int x1, int y1, int newWidth, int newHeight) {
int red00 = (rgb00 >> 16) & 0xFF;
int green00 = (rgb00 >> 8) & 0xFF;
int blue00 = rgb00 & 0xFF;
int red01 = (rgb01 >> 16) & 0xFF;
int green01 = (rgb01 >> 8) & 0xFF;
int blue01 = rgb01 & 0xFF;
int red10 = (rgb10 >> 16) & 0xFF;
int green10 = (rgb10 >> 8) & 0xFF;
int blue10 = rgb10 & 0xFF;
int red11 = (rgb11 >> 16) & 0xFF;
int green11 = (rgb11 >> 8) & 0xFF;
int blue11 = rgb11 & 0xFF;
int red = (int) bilinearInterpolate(red00, red01, red10, red11, x, y, x1, y1, newWidth, newHeight);
int green = (int) bilinearInterpolate(green00, green01, green10, green11, x, y, x1, y1, newWidth, newHeight);
int blue = (int) bilinearInterpolate(blue00, blue01, blue10, blue11, x, y, x1, y1, newWidth, newHeight);
return (red << 16) | (green << 8) | blue;
}
private static double bilinearInterpolate(double f00, double f01, double f10, double f11,
int x, int y, int x1, int y1, int newWidth, int newHeight) {
double widthRatio = (double) (x1 - x) / newWidth;
double heightRatio = (double) (y1 - y) / newHeight;
double f0 = f00 * (1 - widthRatio) + f10 * widthRatio;
double f1 = f01 * (1 - widthRatio) + f11 * widthRatio;
return f0 * (1 - heightRatio) + f1 * heightRatio;
}
}
```
该代码中,`bilinearInterpolation` 方法接收一个原始图像 `image`,以及需要输出的新的宽度和高度 `newWidth` 和 `newHeight`。算法首先创建一个新的 `BufferedImage` 对象 `newImage`,其大小为 `newWidth` 和 `newHeight`。然后,使用双重循环遍历新图像的每个像素位置 `(i, j)`,计算在原图像中对应的坐标 `(x, y)`。然后,根据 `(x, y)` 的值以及原图像上 `(x, y)`、`(x, y1)`、`(x1, y)` 和 `(x1, y1)` 四个像素点的颜色值,使用双线性插值算法计算出 `(i, j)` 上的插值颜色值并设置到 `newImage` 对应位置上。最后返回 `newImage`。
双线性插值计算插值颜色的方法为 `bilinearInterpolate`。该方法接收四个颜色值 `rgb00`、`rgb01`、`rgb10` 和 `rgb11`,以及四个对应像素点 `(x, y)`、`(x, y1)`、`(x1, y)` 和 `(x1, y1)` 的坐标值。方法首先使用位运算和掩码操作提取出每个颜色通道的值,然后根据 `(x, y)`、`(x, y1)`、`(x1, y)` 和 `(x1, y1)` 的值以及新图像的大小计算出对应的权重值。最后,根据权重值使用双线性插值算法计算出 `(x, y)` 像素位置上的插值颜色值。
以上代码是一个简单的双线性插值实现,可以根据需要对其进行调整和优化。
阅读全文
相关推荐



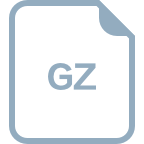










