Dijkstra算法改进
时间: 2024-10-12 20:01:19 浏览: 3
Dijkstra算法是一种用于寻找图中两点之间最短路径的经典贪心算法,但它本身有一些局限性和效率问题。针对这些问题,可以进行一些改进:
1. **优先队列的选择**:原始Dijkstra算法使用邻接矩阵,当图非常大时,操作会变得低效。可以改为使用堆数据结构(如二叉堆),它允许快速插入、删除最小元素,从而加快搜索速度。
2. **权重负值处理**:Dijkstra假设图中所有边都是非负权。如果存在负权重边,可以采用其他算法如Bellman-Ford或SPFA,它们能处理负权边。
3. **增量更新**:当添加新节点或修改边权值时,只对受影响的部分进行重新计算,而非从头开始,这称为A*搜索的启发式版本。
4. **分支限界法**:对于大型网络,可以尝试使用分支限界算法(如IDA*),结合宽度优先搜索,提前剪枝,减少不必要的搜索。
5. **并发优化**:在多线程环境中,可以并行处理多个源点,每个源点独立运行Dijkstra,最后合并结果。
6. **内存优化**:使用迭代加深搜索(IDS),在内存有限的情况下逐步增加搜索深度。
7. **并行Dijkstra**:通过划分顶点集,让多个处理器独立求解,然后合并结果,这是一种并行化的变种。
Dijkstra算法改进的核心是为了应对大规模、实时应用和复杂的图结构,提高其在实际场景中的应用效果和效率。
相关问题
dijkstra 算法改进
Dijkstra算法是解决单源最短路径问题的经典算法,但是随着图问题规模的增大,算法的时间复杂度也会呈指数级增长,因此需要对其进行优化和改进。以下是两种Dijkstra算法的改进思路及对应的Python代码实现:
1.堆优化Dijkstra算法
在Dijkstra算法中,每次需要从未确定最短路径的节点中选取一个距离源点最近的节点进行松弛操作。如果使用线性查找的方式,时间复杂度为O(n^2),如果使用优先队列的方式,时间复杂度为O(mlogn),其中m为边数,n为节点数。但是,如果使用堆优化的方式,可以将时间复杂度降为O(mlogn)。
```python
import heapq
def dijkstra_heap(graph, start):
# 初始化距离字典
dist = {node: float('inf') for node in graph}
dist[start] = 0
# 初始化堆
heap = [(0, start)]
while heap:
# 弹出堆顶元素
(distance, node) = heapq.heappop(heap)
# 如果当前节点已经处理过,跳过
if distance > dist[node]:
continue
# 遍历当前节点的邻居节点
for neighbor, weight in graph[node].items():
# 计算新的距离
new_distance = dist[node] + weight
# 如果新的距离更短,更新距离字典和堆
if new_distance < dist[neighbor]:
dist[neighbor] = new_distance
heapq.heappush(heap, (new_distance, neighbor))
return dist
```
2.双向Dijkstra算法
双向Dijkstra算法是一种优化Dijkstra算法的方式,它从源点和终点同时开始搜索,直到两个搜索路径相遇。这种算法的时间复杂度为O(m^(2/3)),比普通Dijkstra算法的时间复杂度O(mlogn)更快。
```python
def bidirectional_dijkstra(graph, start, end):
# 初始化距离字典
forward_dist = {node: float('inf') for node in graph}
backward_dist = {node: float('inf') for node in graph}
forward_dist[start] = 0
backward_dist[end] = 0
# 初始化前向堆和后向堆
forward_heap = [(0, start)]
backward_heap = [(0, end)]
# 初始化前向节点集合和后向节点集合
forward_visited = set()
backward_visited = set()
while forward_heap and backward_heap:
# 处理前向堆
(forward_distance, forward_node) = heapq.heappop(forward_heap)
forward_visited.add(forward_node)
# 如果当前节点已经在后向节点集合中,返回结果
if forward_node in backward_visited:
return forward_dist[forward_node] + backward_dist[forward_node]
# 遍历当前节点的邻居节点
for neighbor, weight in graph[forward_node].items():
# 计算新的距离
new_distance = forward_dist[forward_node] + weight
# 如果新的距离更短,更新距离字典和堆
if new_distance < forward_dist[neighbor]:
forward_dist[neighbor] = new_distance
heapq.heappush(forward_heap, (new_distance, neighbor))
# 处理后向堆
(backward_distance, backward_node) = heapq.heappop(backward_heap)
backward_visited.add(backward_node)
# 如果当前节点已经在前向节点集合中,返回结果
if backward_node in forward_visited:
return forward_dist[backward_node] + backward_dist[backward_node]
# 遍历当前节点的邻居节点
for neighbor, weight in graph[backward_node].items():
# 计算新的距离
new_distance = backward_dist[backward_node] + weight
# 如果新的距离更短,更新距离字典和堆
if new_distance < backward_dist[neighbor]:
backward_dist[neighbor] = new_distance
heapq.heappush(backward_heap, (new_distance, neighbor))
return float('inf')
```
dijkstra算法的改进
Dijkstra算法是一种经典的单源最短路径算法,但是在实际应用中,我们可能需要对其进行改进以满足特定的需求。以下是几种Dijkstra算法的改进方法:
1. A*算法:A*算法是一种启发式搜索算法,它在Dijkstra算法的基础上增加了一个启发函数,用于估计当前节点到目标节点的距离。这样可以在搜索过程中优先考虑距离目标节点更近的节点,从而提高搜索效率。
2. 堆优化:Dijkstra算法中需要维护一个距离源点最近的未确定节点集合,通常使用优先队列来实现。但是在实际应用中,由于优先队列的常数较大,可能会导致算法效率较低。因此,我们可以使用堆来优化优先队列,从而提高算法效率。
3. 双向Dijkstra算法:双向Dijkstra算法是一种优化的Dijkstra算法,它从源点和目标点同时开始搜索,直到两个搜索路径相遇。这样可以大大减少搜索范围,从而提高算法效率。
相关推荐
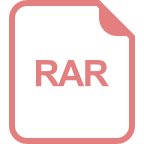
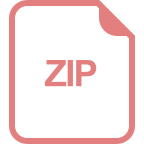













