C++实现获取文件名第九位的字符
时间: 2024-04-10 09:33:04 浏览: 83
要获取文件名的第九位字符,您可以使用C++的标准库函数和字符串操作。以下是一种实现方式:
```cpp
#include <iostream>
#include <string>
char getNinthCharacter(const std::string& filename) {
if (filename.length() >= 9) {
return filename[8];
}
return '\0'; // 如果文件名长度不足9位,则返回空字符
}
int main() {
std::string filename = "example.txt";
char ninthChar = getNinthCharacter(filename);
std::cout << "The ninth character of the filename is: " << ninthChar << std::endl;
return 0;
}
```
在上述代码中,`getNinthCharacter`函数接受文件名作为输入参数,并返回文件名的第九位字符。如果文件名长度小于9位,则返回空字符。
在主函数中,我们示范了如何使用`getNinthCharacter`函数来获取文件名的第九位字符,并将其打印输出。
请注意,上述代码假设文件名是一个字符串类型。如果您从文件路径中提取文件名,请使用适当的方法进行处理,例如使用`std::filesystem`库或自定义字符串操作。
相关问题
C++实现获取文件名第九个字符
你可以使用标准库中的字符串操作函数来获取文件名的第九个字符。以下是一个使用C++的示例代码:
```cpp
#include <iostream>
#include <string>
int main() {
std::string filename = "example.txt";
if (filename.length() >= 9) {
char ninthCharacter = filename[8];
std::cout << "The ninth character of the filename is: " << ninthCharacter << std::endl;
} else {
std::cerr << "The filename is too short to have a ninth character." << std::endl;
}
return 0;
}
```
在上面的代码中,我们假设文件名存储在一个名为`filename`的字符串变量中。我们通过检查字符串的长度来确保它至少包含9个字符。然后,我们可以通过使用索引操作符`[]`来访问第9个字符,索引从0开始,因此第9个字符的索引是8。最后,我们打印出第9个字符。
请注意,上述代码仅演示了如何获取文件名中的第9个字符,并没有涉及实际读取文件的操作。
阅读全文
相关推荐
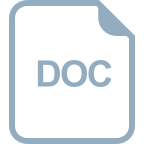
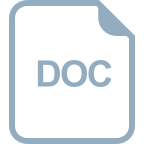
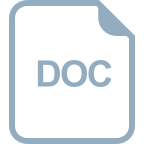
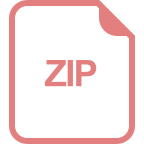
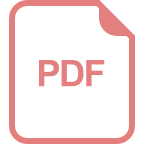
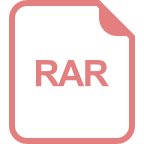
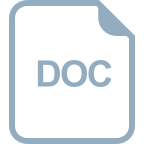
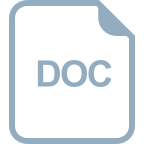
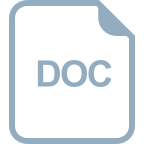
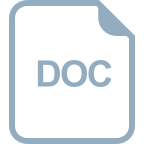
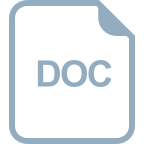
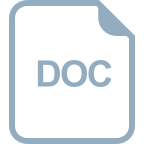
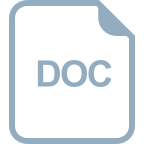
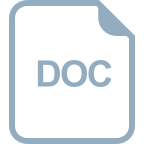
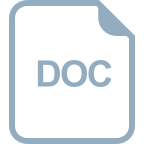
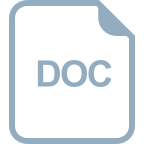
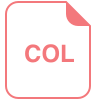
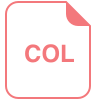