. 使用fork()创建2个子进程,让每个进程在屏幕上输出一个字符。 2. 编写一段程序,实现进程的管道通信。使用pipe()创建一条管道,子进程p1、p2分别向管道各写一句话,父进程从管道读出两个子进程的信息,显示在屏幕上。并输出结果
时间: 2024-11-03 11:14:25 浏览: 12
在Unix/Linux系统中,可以使用`fork()`函数创建新进程,并结合其他系统调用来实现你的需求。以下是两个示例:
1. **使用`fork()`创建子进程输出字符**:
```c
#include <stdio.h>
#include <unistd.h>
#include <sys/wait.h>
int main() {
pid_t pid = fork(); // 创建子进程
if (pid == 0) { // 子进程
char ch = 'A'; // 定义字符
printf("Child process %d is writing '%c'\n", getpid(), ch);
write(1, &ch, sizeof(ch)); // 向标准输出写入字符
exit(0); // 子进程结束
} else { // 父进程
wait(NULL); // 等待子进程结束
printf("Parent process received a character from child.\n");
return 0;
}
}
```
在这个例子中,`fork()`创建了一个子进程,它打印一个字符并将其写入标准输出(通常是屏幕),然后退出。父进程等待子进程结束后继续。
2. **使用`pipe()`实现进程管道通信**:
```c
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
#include <string.h>
#include <fcntl.h>
#define BUFFER_SIZE 1024
int main() {
int pipefd[2]; // 创建管道
pid_t p1, p2;
if (pipe(pipefd) < 0) {
perror("Pipe failed");
return -1;
}
p1 = fork();
if (p1 == 0) { // 子进程 p1
close(pipefd[0]); // 关闭读端
char message[] = "Hello from p1";
write(pipefd[1], message, strlen(message) + 1);
close(pipefd[1]);
exit(0);
} else {
close(pipefd[1]); // 父进程关闭写端
p2 = fork();
if (p2 == 0) { // 子进程 p2
close(pipefd[0]); // 关闭父进程的读端
char buffer[BUFFER_SIZE];
read(pipefd[0], buffer, BUFFER_SIZE);
printf("Received from p1: %s\n", buffer);
exit(0);
} else { // 父进程
close(pipefd[0]); // 关闭子进程的读端
wait(NULL); // 等待子进程结束
printf("Parent received messages from both children.\n");
// Read from p2 and print
read(pipefd[1], buffer, BUFFER_SIZE);
printf("Received from p2: %s\n", buffer);
close(pipefd[1]);
return 0;
}
}
}
```
这里首先创建了管道,然后通过`fork()`创建两个子进程p1和p2。p1写入消息,p2读取并打印,最后父进程再读取一次。注意,为了实现双向通信,每个进程需要关闭不需要的那一端的管道描述符。
阅读全文
相关推荐
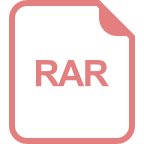
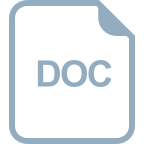
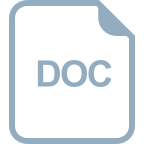
















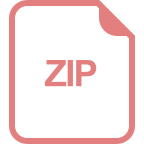