java获取导入excel模版链接数据库
时间: 2025-01-05 16:46:39 浏览: 15
在Java中获取导入Excel模板并链接数据库的步骤可以分为以下几个部分:
### 1. 导入Excel文件
首先,你需要使用Apache POI库来读取Excel文件。Apache POI是一个强大的Java库,用于读写Microsoft Office文档。
```java
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
public class ExcelReader {
public static void main(String[] args) {
String excelFilePath = "path/to/your/excel/file.xlsx";
FileInputStream fis = null;
Workbook workbook = null;
try {
fis = new FileInputStream(new File(excelFilePath));
workbook = new XSSFWorkbook(fis);
Sheet sheet = workbook.getSheetAt(0);
for (Row row : sheet) {
for (Cell cell : row) {
switch (cell.getCellType()) {
case STRING:
System.out.print(cell.getStringCellValue() + "\t");
break;
case NUMERIC:
System.out.print(cell.getNumericCellValue() + "\t");
break;
case BOOLEAN:
System.out.print(cell.getBooleanCellValue() + "\t");
break;
default:
System.out.print("-\t");
}
}
System.out.println();
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (workbook != null) workbook.close();
if (fis != null) fis.close();
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
}
```
### 2. 链接数据库
接下来,你需要使用JDBC来链接数据库。以下是一个简单的示例,展示了如何链接MySQL数据库并插入数据。
```java
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class DatabaseConnector {
private static final String URL = "jdbc:mysql://localhost:3306/your_database";
private static final String USER = "your_username";
private static final String PASSWORD = "your_password";
public static Connection getConnection() throws SQLException {
return DriverManager.getConnection(URL, USER, PASSWORD);
}
public static void insertData(String data) {
String sql = "INSERT INTO your_table (column_name) VALUES (?)";
try (Connection conn = getConnection();
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setString(1, data);
pstmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
```
### 3. 结合Excel读取和数据库插入
最后,你需要将Excel读取和数据库插入结合起来。以下是一个简单的示例:
```java
public class ExcelToDatabase {
public static void main(String[] args) {
String excelFilePath = "path/to/your/excel/file.xlsx";
FileInputStream fis = null;
Workbook workbook = null;
try {
fis = new FileInputStream(new File(excelFilePath));
workbook = new XSSFWorkbook(fis);
Sheet sheet = workbook.getSheetAt(0);
for (Row row : sheet) {
Cell cell = row.getCell(0); // Assuming data is in the first column
if (cell != null) {
String data = cell.getStringCellValue();
DatabaseConnector.insertData(data);
}
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (workbook != null) workbook.close();
if (fis != null) fis.close();
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
}
```
###
阅读全文
相关推荐












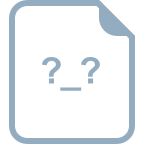





