51单片机通过串口把Lcd1602的数据显示在电脑上
时间: 2024-04-18 18:25:38 浏览: 34
你可以通过使用51单片机的串口通信功能将LCD1602的数据显示在电脑上。首先,你需要连接51单片机和LCD1602模块。通常,LCD1602模块包含16x2个字符的显示屏和一个控制芯片(如HD44780),它们通过并行接口与单片机连接。
接下来,你需要配置51单片机的串口通信功能。通过设置波特率、数据位、停止位和校验位等参数,你可以确保单片机和电脑之间的正确数据传输。然后,编写单片机程序,使用串口发送LCD1602的数据到电脑。
在电脑上,你需要使用串口调试助手或者其他串口通信软件来接收和显示从单片机发送过来的数据。打开串口调试助手,并选择正确的串口号和波特率。当单片机发送数据时,你将在串口调试助手的接收区域看到相应的数据显示。
总结起来,你需要进行以下步骤:
1. 连接51单片机和LCD1602模块。
2. 配置51单片机的串口通信参数。
3. 编写单片机程序,将LCD1602的数据通过串口发送到电脑。
4. 在电脑上使用串口调试助手或其他串口通信软件来接收和显示数据。
希望以上信息对你有所帮助!如果还有其他问题,请随时提问。
相关问题
51单片机通过串口控制lcd1602来改变时钟的代码
以下是一个简单的51单片机程序,通过串口控制LCD1602来改变时钟:
```c
#include <reg52.h>
#include <stdio.h>
#define LCD1602_DB P0
sbit LCD1602_RS = P2^0;
sbit LCD1602_RW = P2^1;
sbit LCD1602_E = P2^2;
void delay(unsigned int xms) // 延时函数
{
unsigned int i, j;
for(i=xms; i>0; i--)
for(j=112; j>0; j--);
}
void LCD1602_WriteCommand(unsigned char com) // 写命令
{
LCD1602_RS = 0;
LCD1602_RW = 0;
LCD1602_DB = com;
LCD1602_E = 1;
delay(5);
LCD1602_E = 0;
}
void LCD1602_WriteData(unsigned char dat) // 写数据
{
LCD1602_RS = 1;
LCD1602_RW = 0;
LCD1602_DB = dat;
LCD1602_E = 1;
delay(5);
LCD1602_E = 0;
}
void LCD1602_Init() // 初始化LCD1602
{
LCD1602_WriteCommand(0x38);
LCD1602_WriteCommand(0x0c);
LCD1602_WriteCommand(0x06);
LCD1602_WriteCommand(0x01);
}
void LCD1602_ShowString(unsigned char x, unsigned char y, unsigned char *str) // 在指定位置显示字符串
{
unsigned char i = 0;
if(x<16) // x不能大于16
{
if(y) // y=1,第二行
LCD1602_WriteCommand(0xc0+x);
else // y=0,第一行
LCD1602_WriteCommand(0x80+x);
while(str[i]!='\0') // 写入每个字符
{
LCD1602_WriteData(str[i]);
i++;
}
}
}
void main()
{
unsigned char hour = 0, minute = 0; // 定义变量
unsigned char str[16];
LCD1602_Init(); // 初始化LCD1602
while(1)
{
if(RI) // 如果接收到数据
{
RI = 0;
hour = SBUF; // 读取小时
while(!RI); // 等待接收到数据
RI = 0;
minute = SBUF; // 读取分钟
sprintf(str, "%02d:%02d", hour, minute); // 格式化字符串
LCD1602_ShowString(0, 0, str); // 在LCD1602上显示
}
}
}
```
这个程序通过串口接收小时和分钟,然后格式化字符串并在LCD1602上显示。你可以根据需要修改代码以实现更多功能。
基于c51单片机实现通过电脑串口向LCD1602发送数据并显示的代码
以下是基于C51单片机实现通过电脑串口向LCD1602发送数据并显示的代码:
```c
#include <reg51.h>
#define LCD_DB P0
sbit LCD_RS = P2^0;
sbit LCD_EN = P2^1;
void delay(unsigned int i)
{
while(i--);
}
void LCD_init()
{
delay(15000);
LCD_RS = 0;
LCD_EN = 0;
LCD_DB = 0x38;
LCD_EN = 1;
LCD_EN = 0;
delay(5000);
LCD_DB = 0x0C;
LCD_EN = 1;
LCD_EN = 0;
delay(5000);
LCD_DB = 0x01;
LCD_EN = 1;
LCD_EN = 0;
delay(5000);
LCD_DB = 0x06;
LCD_EN = 1;
LCD_EN = 0;
}
void LCD_write_cmd(unsigned char cmd)
{
LCD_RS = 0;
LCD_EN = 0;
LCD_DB = cmd;
LCD_EN = 1;
LCD_EN = 0;
}
void LCD_write_data(unsigned char dat)
{
LCD_RS = 1;
LCD_EN = 0;
LCD_DB = dat;
LCD_EN = 1;
LCD_EN = 0;
}
void LCD_write_string(unsigned char x, unsigned char y, unsigned char *s)
{
unsigned char i = 0;
if(x < 16)
{
LCD_write_cmd(0x80 + y*0x40 + x);
}
else
{
LCD_write_cmd(0x80 + y*0x40 + x - 16);
}
while(s[i] != '\0')
{
LCD_write_data(s[i]);
i++;
}
}
void UART_init()
{
TMOD |= 0x20;
TH1 = 0xFD;
TL1 = 0xFD;
TR1 = 1;
SM0 = 0;
SM1 = 1;
REN = 1;
EA = 1;
ES = 1;
}
void UART_interrupt() interrupt 4
{
if(RI)
{
RI = 0;
LCD_write_data(SBUF);
}
if(TI)
{
TI = 0;
}
}
void main()
{
LCD_init();
UART_init();
while(1)
{
LCD_write_string(0, 0, "Serial Port Test");
LCD_write_string(0, 1, "Data: ");
}
}
```
其中,使用P0口作为LCD1602的数据口,使用P2.0和P2.1口作为LCD1602的控制口,使用Timer1作为波特率发生器,使用中断实现接收和发送数据。在UART_interrupt()函数中,RI表示接收到数据,TI表示发送数据完成。在主函数中,通过LCD_write_string()函数向LCD1602上显示字符串。
相关推荐
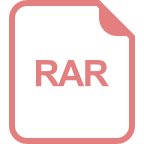
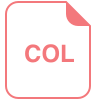
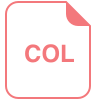










