tf.unstack
时间: 2023-12-11 15:45:21 浏览: 30
tf.unstack 函数是 TensorFlow 中的一个操作,用于将张量沿着指定维度进行拆分。它的语法如下:
```python
tf.unstack(value, axis=0, num=None, name='unstack')
```
参数说明:
- `value`:需要拆分的张量。
- `axis`:指定要沿着的维度。默认为 0。
- `num`:拆分后的张量数量。如果未指定,则默认为 value.shape[axis]。
- `name`:操作的名称。
返回值:
一个包含拆分后张量的列表。
例如,假设有一个 shape 为 (3, 4) 的张量,通过 `tf.unstack` 操作可以将其沿着维度 0 拆分成 3 个大小为 (4,) 的张量。
```python
import tensorflow as tf
x = tf.constant([[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12]])
unstacked = tf.unstack(x, axis=0)
print(unstacked)
```
输出结果为:
```
相关问题
output = tf.keras.rnn.static_rnn(cell, seqTimeSteps, dtype = tf.float64)
在 TensorFlow 2.x 中,`tf.keras.rnn.static_rnn` 函数已经被弃用,如果想要在 TensorFlow 2.x 中使用 RNN 网络,可以使用 `tf.keras.layers.RNN` 类,或者使用 `tf.keras.layers.SimpleRNN`、`tf.keras.layers.LSTM`、`tf.keras.layers.GRU` 等层来构建 RNN 网络。
如果你想使用类似 `tf.keras.rnn.static_rnn` 的函数,可以使用 `tf.compat.v1.nn.static_rnn` 函数来代替,如下所示:
```python
import tensorflow as tf
# 定义 RNN 网络结构
cell = tf.compat.v1.nn.rnn_cell.BasicRNNCell(num_units=128)
inputs = tf.random.normal(shape=(32, 10, 10))
seqTimeSteps = tf.unstack(inputs, axis=1)
outputs, state = tf.compat.v1.nn.static_rnn(cell, seqTimeSteps, dtype=tf.float64)
# 定义模型
model = tf.keras.Model(inputs=inputs, outputs=outputs)
# 编译模型
model.compile(optimizer='adam', loss='mse', metrics=['mae'])
# 训练模型
x_train = tf.random.normal(shape=(32, 10, 10))
y_train = tf.random.normal(shape=(32, 10, 128))
model.fit(x_train, y_train, batch_size=8, epochs=10)
```
在上面的代码中,我们首先使用 `tf.compat.v1.nn.rnn_cell.BasicRNNCell` 定义了一个基础的 RNN 单元,然后使用 `tf.compat.v1.nn.static_rnn` 函数对输入数据进行处理。最后使用 `tf.keras.Model` 定义了一个完整的模型,并使用 `model.compile` 和 `model.fit` 进行模型的编译和训练。需要注意的是,在使用 `tf.compat.v1.nn.static_rnn` 函数时,需要将输入数据转换为 `list` 类型的张量,并且需要指定 `dtype` 参数。
tf.keras.rnn.static_rnn怎么适配tensorflow 2.x版本
在 TensorFlow 2.x 版本中,`tf.keras` 模块已经被大量改进,包括 `tf.keras.layers.RNN` 类替代了 `tf.keras.rnn` 模块中的 `static_rnn` 函数。因此,在 TensorFlow 2.x 版本中,如果要使用 `static_rnn` 函数,需要使用 `tf.compat.v1` 模块进行适配。下面是一个例子:
```python
import tensorflow as tf
# 定义 RNN 网络结构
cell = tf.compat.v1.nn.rnn_cell.BasicRNNCell(num_units=128)
inputs = tf.keras.Input(shape=(None, 10))
outputs, state = tf.compat.v1.nn.static_rnn(cell, tf.unstack(inputs, axis=1), dtype=tf.float32)
# 定义模型
model = tf.keras.Model(inputs=inputs, outputs=outputs)
# 编译模型
model.compile(optimizer='adam', loss='mse', metrics=['mae'])
# 训练模型
x_train = tf.random.normal(shape=(32, 10, 10))
y_train = tf.random.normal(shape=(32, 10, 128))
model.fit(x_train, y_train, batch_size=8, epochs=10)
```
在上面的代码中,我们首先使用 `tf.compat.v1.nn.rnn_cell.BasicRNNCell` 定义了一个基础的 RNN 单元,然后使用 `tf.compat.v1.nn.static_rnn` 函数对输入数据进行处理。最后使用 `tf.keras.Model` 定义了一个完整的模型,并使用 `model.compile` 和 `model.fit` 进行模型的编译和训练。需要注意的是,在使用 `tf.compat.v1.nn.static_rnn` 函数时,需要将输入数据转换为 `list` 类型的张量,并且需要指定 `dtype` 参数。
相关推荐
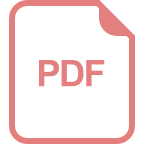
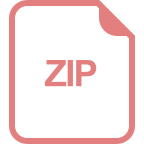
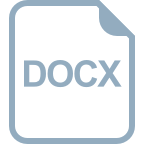












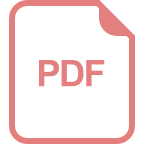