c语言怎么将2进制的utf-8格式解码
时间: 2024-09-08 07:00:49 浏览: 18
在C语言中,将UTF-8格式的二进制数据解码成字符需要通过遍历字节序列并处理每个编码单元。UTF-8是一种变长的编码方式,用于表示Unicode字符集中的字符。对于每个字符,它可能会由1到4个连续的字节组成。
以下是一个简单的步骤说明:
1. **预处理**:
- 定义一个函数,比如`decode_utf8()`,接收一个指向UTF-8字节数组的指针和长度作为输入参数。
2. **循环解析**:
- 使用一个for循环,从第一个字节开始,每次迭代检查当前字节数组中的前几个字节。
- 对于每个编码单元,判断其第一字节:
- 如果它是0到127范围内的值(1个字节),直接读取这1个字节作为ASCII字符。
- 如果是192到223范围内的值(2个字节),读取接下来的两个字节作为高两位和低六位的数据,合并后转换为对应的Unicode点。
- 对于224到239范围(3个字节)、240到247范围(4个字节)的情况,同样道理,逐次读取后续字节并组合。
3. **构建字符**:
- 每获取一组字节后,使用`unichr()`函数(或在C11及以上版本使用`u_char_to_u32()`,然后转换为对应的`char`类型的字符。
4. **字符串连接**:
- 将所有解码后的字符添加到结果字符串中。
5. **返回结果**:
- 当所有字节都处理完后,返回包含解码字符的字符串。
```c
#include <stdio.h>
#include <string.h>
// 示例函数,假设输入的字节是unsigned char类型
char *decode_utf8(const unsigned char* bytes, size_t length) {
char *decoded = malloc(length);
int decoded_index = 0;
for (size_t i = 0; i < length; ++i) {
unsigned char byte = bytes[i];
if (byte <= 0x7F) { // 1字节字符
decoded[decoded_index++] = byte;
} else if (byte >= 0xC2 && byte <= 0xDF) { // 2字节字符
decoded[decoded_index++] = ((byte & 0x1F) << 6) | (bytes[++i] & 0x3F);
} else if (byte >= 0xE0 && byte <= 0xEF) { // 3字节字符
decoded[decoded_index++] = ((byte & 0x0F) << 12) | ((bytes[++i] & 0x3F) << 6) | (bytes[++i] & 0x3F);
} else if (byte >= 0xF0 && byte <= 0xF4) { // 4字节字符
decoded[decoded_index++] = ((byte & 0x07) << 18) | ((bytes[++i] & 0x3F) << 12) | ((bytes[++i] & 0x3F) << 6) | (bytes[++i] & 0x3F);
}
}
decoded[decoded_index] = '\0'; // 添加字符串结束符
return decoded;
}
int main() {
// 实际使用时替换为实际的UTF-8字节数据
const unsigned char utf8_bytes[] = {0xED, 0xA0, 0x80, ...}; // 示例,实际应为完整的UTF-8编码
size_t length = strlen((const char*)utf8_bytes); // 获取字节数
char *decoded_str = decode_utf8(utf8_bytes, length);
printf("Decoded string: %s\n", decoded_str);
free(decoded_str); // 注意释放动态分配的内存
return 0;
}
```
相关推荐
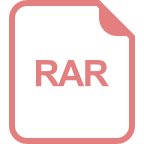
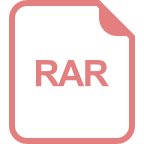
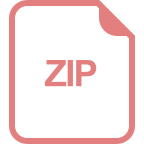














