拖动条形彩色条上的小球,小球落在不同颜色上时,展示出不同颜色对应的不同信息,如何实现
时间: 2024-09-24 11:28:21 浏览: 21
实现这个功能通常涉及到前端开发,特别是JavaScript、HTML和CSS。以下是基本步骤:
1. HTML结构:创建一个包含彩色条和小球的容器。可以使用`<div>`元素表示彩色条,`<span>`元素作为小球。
```html
<div id="color-slider" class="slider">
<span class="ball"></span>
</div>
```
2. CSS样式:设置彩色条的外观和小球的位置,并为不同颜色添加样式。例如,你可以给每个颜色块设置背景色。
```css
.slider {
width: 300px;
height: 50px;
position: relative;
}
.ball {
position: absolute;
top: 50%;
transform: translateY(-50%);
}
```
3. JavaScript交互:通过JavaScript监听`mousedown`、`mousemove`和`mouseup`事件来跟踪鼠标移动。当小球接触到不同颜色时,改变其内部的DOM内容或CSS类,显示对应的信息。
```javascript
const slider = document.getElementById('color-slider');
const ball = document.querySelector('.ball');
let currentColor;
slider.addEventListener('mousedown', startDrag);
slider.addEventListener('mouseup', endDrag);
function startDrag(e) {
ball.style.position = 'absolute';
[x, y] = getMousePosition(slider, e);
updateBallPosition(x, y);
}
function endDrag() {
ball.style.position = 'relative';
}
function updateBallPosition(x, y) {
// 根据x坐标计算当前的颜色区域
// 更新ball元素的内容(如innerText)或类名,显示对应信息
showInfo(currentColor);
}
function getMousePosition(element, event) {
return [
event.clientX - element.getBoundingClientRect().left,
event.clientY - element.getBoundingClientRect().top
];
}
// 示例:获取颜色并显示信息
function showInfo(color) {
if (currentColor !== color) {
// 更新或切换颜色对应的文本或显示
currentColor = color;
ball.textContent = `当前颜色: ${color}`;
}
}
// 需要自定义的部分:计算当前颜色并关联到实际的数据映射
function getColorAtPoint(x, y) {
// 这里根据滑块的结构和颜色分布实现
// 返回对应的颜色名称
}
```
相关推荐
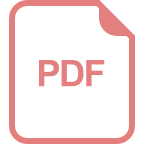
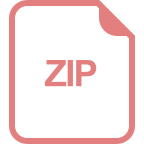
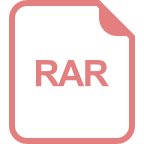














