(一)1.使用List集合存储10个学生信息(建学生类)。 学生信息:姓名,年龄,成绩。 统计所有姓“张”的同学的平均成绩
时间: 2024-10-18 08:10:06 浏览: 32
首先,你需要创建一个名为`Student`的学生类,包含姓名、年龄和成绩属性。然后使用`List<Student>`来存储10个学生的实例。假设`Student`类的结构如下:
```java
public class Student {
private String name;
private int age;
private double score;
// 构造函数,getters和setters...
public Student(String name, int age, double score) {
this.name = name;
this.age = age;
this.score = score;
}
// 获取和设置方法...
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public int getAge() { return age; }
public void setAge(int age) { this.age = age; }
public double getScore() { return score; }
public void setScore(double score) { this.score = score; }
}
```
接下来,你可以创建一个列表来存储学生,并统计姓“张”的学生平均成绩:
```java
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<Student> students = new ArrayList<>();
for (int i = 0; i < 10; i++) {
String name = "张" + (i+1); // 假设前10个学生都姓张
int age = i + 18; // 假设年龄从18岁开始递增
double score = i * 10; // 假设分数递增
students.add(new Student(name, age, score));
}
double totalScore = 0;
int count = 0;
for (Student student : students) {
if (student.getName().startsWith("张")) {
totalScore += student.getScore();
count++;
}
}
if (count > 0) {
double averageScore = totalScore / count;
System.out.println("姓张的同学平均成绩为:" + averageScore);
} else {
System.out.println("没有找到姓张的同学");
}
}
}
```
在这个例子中,我们遍历学生列表,如果发现学生的姓名以“张”开头,就累加他们的成绩并增加计数器。最后,计算平均值并打印出来。
阅读全文
相关推荐



















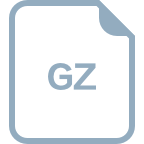