用c语言创建一个单链表来储存成绩,并能输出成绩的最高分和平均分
时间: 2024-10-11 10:13:47 浏览: 32
在C语言中,我们可以创建一个结构体来存储学生的姓名和分数,然后用一个单链表来存储这个结构体实例。这里是一个简单的示例,首先定义链表节点结构,然后创建链表、插入数据、找到最高分并计算平均分:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct Node {
char name[50];
int score;
struct Node* next;
} StudentNode;
// 创建链表头指针
StudentNode* head = NULL;
// 插入新节点
void insertStudent(char* name, int score) {
StudentNode* newNode = (StudentNode*)malloc(sizeof(StudentNode));
if (newNode != NULL) {
strcpy(newNode->name, name);
newNode->score = score;
newNode->next = head;
head = newNode;
}
}
// 打印链表中所有学生的成绩
void printScores() {
StudentNode* temp = head;
while (temp != NULL) {
printf("学生:%s, 分数:%d\n", temp->name, temp->score);
temp = temp->next;
}
}
// 寻找最高分
int findMaxScore() {
int maxScore = INT_MIN;
StudentNode* temp = head;
while (temp != NULL) {
if (temp->score > maxScore) {
maxScore = temp->score;
}
temp = temp->next;
}
return maxScore;
}
// 计算平均分
double calculateAverage() {
int sum = 0;
int count = 0;
StudentNode* temp = head;
while (temp != NULL) {
sum += temp->score;
count++;
temp = temp->next;
}
return (double)sum / count;
}
int main() {
// 插入一些学生数据
insertStudent("张三", 90);
insertStudent("李四", 85);
insertStudent("王五", 95);
// 打印成绩
printScores();
// 输出最高分和平均分
int max = findMaxScore();
double avg = calculateAverage();
printf("最高分:%d\n", max);
printf("平均分:%.2f\n", avg);
return 0;
}
```
阅读全文
相关推荐
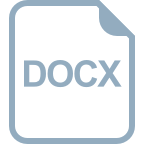
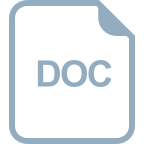















