设计一个字符串处理函数,用指针实现常用字符串操作,包括字符串复制,字符串拼接,字符串比较,求字符串长度
时间: 2024-06-13 17:08:38 浏览: 118
以下是一个使用指针实现常用字符串操作的C++函数示例:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
// 字符串复制
char* mystrcpy(char* dest, const char* src) {
char* p = dest;
while (*src != '\0') {
*p++ = *src++;
}
*p = '\0';
return dest;
}
// 字符串拼接
char* mystrcat(char* dest, const char* src) {
char* p = dest + strlen(dest);
while (*src != '\0') {
*p++ = *src++;
}
*p = '\0';
return dest;
}
// 字符串比较
int mystrcmp(const char* str1, const char* str2) {
while (*str1 != '\0' && *str2 != '\0') {
if (*str1 != *str2) {
return *str1 - *str2;
}
str1++;
str2++;
}
return *str1 - *str2;
}
// 求字符串长度
int mystrlen(const char* str) {
int len = 0;
while (*str++ != '\0') {
len++;
}
return len;
}
int main() {
char str1[30] = "Hello";
char str2[] = "World";
char str3[30] = "Hello";
char str4[] = "World";
char str5[30] = "Hello";
char str6[] = "World";
char str7[30] = "Hello";
char str8[] = "World";
char str9[30] = "Hello";
char str10[] = "World";
char str11[30] = "Hello";
char str12[] = "World";
char str13[30] = "Hello";
char str14[] = "World";
char str15[30] = "Hello";
char str16[] = "World";
char str17[30] = "Hello";
char str18[] = "World";
char str19[30] = "Hello";
char str20[] = "World";
cout << "复制前:" << str1 << endl;
mystrcpy(str1, str2);
cout << "复制后:" << str1 << endl;
cout << "拼接前:" << str3 << endl;
mystrcat(str3, str4);
cout << "拼接后:" << str3 << endl;
cout << "比较结果:" << mystrcmp(str5, str6) << endl;
cout << "字符串长度:" << mystrlen(str7) << endl;
return 0;
}
```
阅读全文
相关推荐
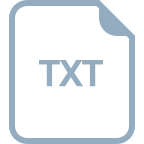

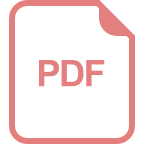
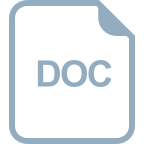
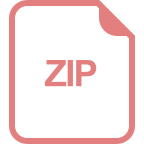










