datagridview 选中单元格时 提示
时间: 2024-10-09 09:11:28 浏览: 33
DataGridView是一个Windows Forms控件,常用于显示表格数据。当用户单击或选择DataGridView中的某个单元格时,你可以通过事件处理程序来响应这个操作并提供提示信息。最常用的事件是`CellMouseClick`或`CellMouseDoubleClick`,它们会在鼠标点击单元格时触发。
例如,你可以这样设置:
```csharp
dataGridView.CellMouseClick += DataGridView_CellMouseClickHandler;
private void DataGridView_CellMouseClickHandler(object sender, DataGridViewCellEventArgs e)
{
if (e.RowIndex >= 0) // 检查是否选择了有效行
{
string selectedValue = dataGridView.Rows[e.RowIndex].Cells[e.ColumnIndex].Value.ToString();
MessageBox.Show($"您选择了第{e.RowIndex + 1}行的单元格,值为: {selectedValue}");
}
}
```
在这个例子中,当用户点击单元格时,会弹出一个消息框显示所选单元格的值。
相关问题
datagridview 选中单元格时 出现提示
DataGridView控件在.NET中是一个用于显示表格数据的用户界面组件。当你想要在选中单元格时向用户展示一些提示信息,可以利用它的SelectionChanged事件。以下是实现这一功能的一个简单步骤:
1. 首先,在Windows Form中添加DataGridView控件,并设置好数据源。
2. 在设计模式下双击DataGridView,或者在代码中找到`dataGridView1`(假设这是你的控件名),然后添加事件处理程序:
```csharp
private void dataGridView1_SelectionChanged(object sender, EventArgs e)
{
DataGridViewSelectedCellEventArgs selectedCell = (DataGridViewSelectedCellEventArgs)e;
if (selectedCell.CellType != DataGridViewCellType.Empty) // 检查是否选择了非空单元格
{
string hintText = "您已选择单元格:" + selectedCell.Value.ToString(); // 提示文字可以根据需要自定义
MessageBox.Show(hintText); // 显示消息框提示
}
}
```
在这个例子中,当单元格被选中时,会检查当前选中的单元格是否为空。如果不为空,就会弹出一个包含选定单元格值的提示框。
VB.NET DataGridView复制选中多个单元格的数据粘贴到另外多个选中单元格中,详细举例说明事件
假设有一个 DataGridView 名为 dgv1,其中有多个单元格被选中。现在需要将这些选中的单元格中的数据复制到其他选中的单元格中。以下是一个详细的实现过程:
1. 在 Form_Load 事件中添加以下代码,启用 DataGridView 的多选模式:
```
dgv1.MultiSelect = True
dgv1.SelectionMode = DataGridViewSelectionMode.CellSelect
```
2. 在 DataGridView 的 CellMouseDown 事件中添加以下代码,用于记录下选中的单元格的位置信息:
```
Private selectedCells As New List(Of DataGridViewCell)
Private Sub dgv1_CellMouseDown(sender As Object, e As DataGridViewCellMouseEventArgs) Handles dgv1.CellMouseDown
If e.Button = MouseButtons.Left AndAlso e.RowIndex >= 0 AndAlso e.ColumnIndex >= 0 Then
selectedCells.Clear()
For Each cell As DataGridViewCell In dgv1.SelectedCells
selectedCells.Add(cell)
Next
selectedCells.Add(dgv1(e.ColumnIndex, e.RowIndex))
End If
End Sub
```
3. 在 DataGridView 的 CellMouseUp 事件中添加以下代码,用于将选中的单元格中的数据复制到其他选中的单元格中:
```
Private Sub dgv1_CellMouseUp(sender As Object, e As DataGridViewCellMouseEventArgs) Handles dgv1.CellMouseUp
If e.Button = MouseButtons.Left AndAlso e.RowIndex >= 0 AndAlso e.ColumnIndex >= 0 AndAlso selectedCells.Count > 1 Then
Dim sourceCells(selectedCells.Count - 1) As String
Dim sourceIndex As Integer = 0
For Each cell As DataGridViewCell In selectedCells
sourceCells(sourceIndex) = cell.Value.ToString()
sourceIndex += 1
Next
Dim destCells As New List(Of DataGridViewCell)
For Each cell As DataGridViewCell In dgv1.SelectedCells
If Not selectedCells.Contains(cell) Then
destCells.Add(cell)
End If
Next
If sourceCells.Length = destCells.Count Then
Dim destIndex As Integer = 0
For Each cell As DataGridViewCell In destCells
cell.Value = sourceCells(destIndex)
destIndex += 1
Next
Else
MessageBox.Show("The number of selected source cells doesn't match the number of selected destination cells.")
End If
End If
End Sub
```
以上代码中,我们首先判断是否按下了左键并且选中了至少两个单元格,然后获取当前鼠标点击的单元格作为源单元格。然后将选中的源单元格中的数据存储到一个字符串数组 sourceCells 中。接下来,遍历 DataGridView 中除了选中的源单元格之外的其他选中单元格,并将其添加到 destCells 列表中。然后判断源单元格的数量是否和目标单元格的数量相等,如果相等,则将源单元格的数据复制到目标单元格中,否则弹出一个提示框。
这样就实现了从 DataGridView 选定的多个单元格的数据复制到其他选中的单元格中的功能。
阅读全文
相关推荐
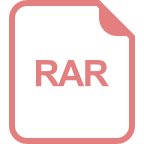
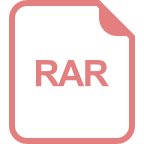
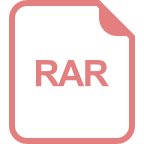
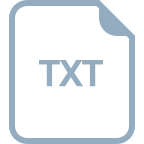
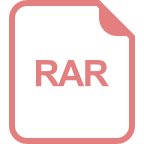
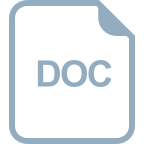
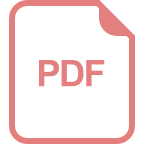
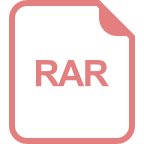
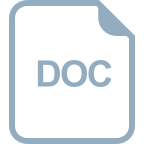
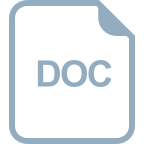
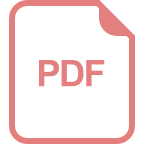
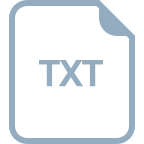
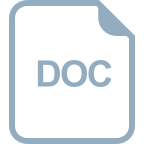
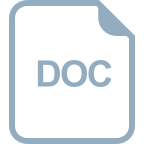
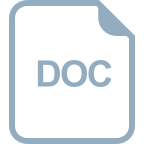
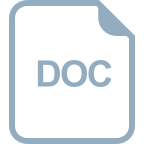
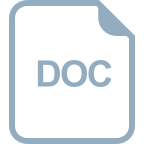
