<template> <div>子组件:</div> <input type="text" v-model="msg"> <h1>{{ msg }}</h1> <h1>{{age}}</h1> </template> <script setup lang=ts> // import { defineEmits } from "vue"; const emit = defineEmits(["updata:msg", "updata:age"]); const props = defineProps({ msg:{ type:String, default:"", }, age:{ type:Number, default:0, } }) </script>
时间: 2024-04-15 12:29:48 浏览: 120
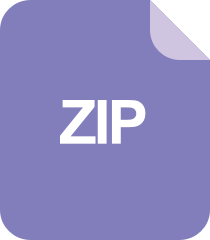
HTML页面不认<script type="text/template">标签,怎么办?

It seems like you're trying to create a Vue.js component with TypeScript using the `<script setup>` syntax. In your code, you have defined a component with a template that includes an input field and two `<h1>` tags to display the values of `msg` and `age` props.
To make your component work properly, you to make a few adjustments. Here's the updated code:
```html
<template>
<div>
<div>子组件:</div>
<input type="text" v-model="localMsg">
<h1>{{ localMsg }}</h1>
<h1>{{ localAge }}</h1>
</div>
</template>
<script setup lang="ts">
import { defineProps, defineEmits } from 'vue';
const props = defineProps({
msg: {
type: String,
default: '',
},
age: {
type: Number,
default: 0,
},
});
const emit = defineEmits(['update:msg', 'update:age']);
let localMsg = props.msg;
let localAge = props.age;
// Watch for changes in localMsg and emit the update event
watch(() => localMsg, (newValue) => {
emit('update:msg', newValue);
});
// Watch for changes in localAge and emit the update event
watch(() => localAge, (newValue) => {
emit('update:age', newValue);
});
</script>
```
In the updated code:
1. We import `defineProps` and `defineEmits` from the Vue Composition API.
2. We define `props` using `defineProps` with the specified types and default values.
3. We define `emit` using `defineEmits` to handle the custom events.
4. We initialize `localMsg` and `localAge` with the prop values.
5. We use the `watch` function to listen to changes in `localMsg` and `localAge`. When a change occurs, we emit the corresponding update event.
With these changes, your component should now properly handle the two-way data binding between the input field and the props `msg` and `age`.
阅读全文
相关推荐
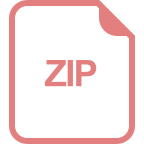
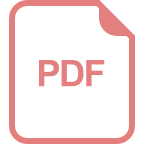




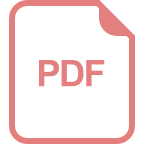
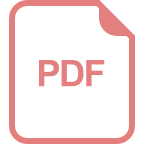
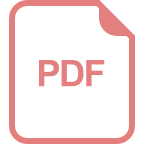
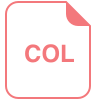
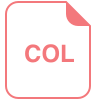
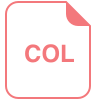
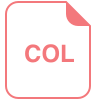
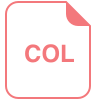
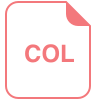
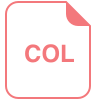
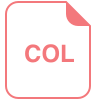
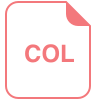