数据结构C语言第2版队列的创建及基本操作的实现
时间: 2024-10-13 08:19:09 浏览: 33
在 C 语言中,我们可以使用数组或链表来实现队列的数据结构。这里我会简单讲解基于数组的队列实现,因为数组操作相对简单直观。
**一、队列的创建**
首先,我们需要一个数组作为存储空间,以及两个指针 `front` 和 `rear` 分别表示队首和队尾的位置。创建时,初始化这两个指针为0,表示队列为空。
```c
typedef struct {
int capacity; // 队列容量
int size; // 当前元素数量
int* data; // 存储数据的数组
} Queue;
Queue* createQueue(int capacity) {
Queue* queue = (Queue*)malloc(sizeof(Queue));
if (!queue) {
printf("内存分配失败");
return NULL;
}
queue->capacity = capacity;
queue->size = 0;
queue->data = (int*)malloc(capacity * sizeof(int));
if (!queue->data) {
free(queue);
return NULL;
}
queue->front = queue->rear = 0;
return queue;
}
```
**二、基本操作实现**
1. **入队 (`enqueue`)**:
将元素添加到队尾。如果队列已满,则需要先扩容。
```c
void enqueue(Queue* queue, int value) {
if (queue->size == queue->capacity) {
// 扩容
queue->capacity *= 2;
void* new_data = realloc(queue->data, queue->capacity * sizeof(int));
if (!new_data) {
printf("扩容失败");
return;
}
queue->data = (int*)new_data;
}
queue->data[queue->rear] = value;
queue->rear = (queue->rear + 1) % queue->capacity; // 循环索引
queue->size++;
}
```
2. **出队 (`dequeue`)**:
如果队列非空,删除并返回队首元素,然后更新队首位置。
```c
int dequeue(Queue* queue) {
if (queue->size == 0) {
printf("队列为空");
return -1;
}
int value = queue->data[queue->front];
queue->front = (queue->front + 1) % queue->capacity;
queue->size--;
return value;
}
```
3. **查看队头/队尾元素**:
可以通过直接访问 `queue->data[front]` 或 `queue->data[rear]` 来查看队头或队尾元素,无需实际出队/入队操作。
阅读全文
相关推荐
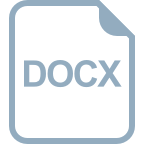
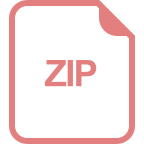
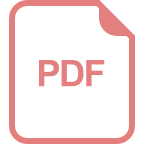
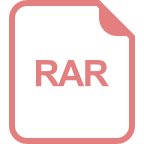
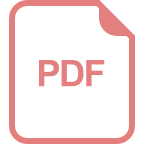
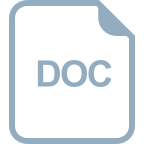
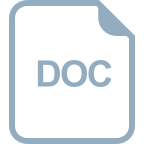
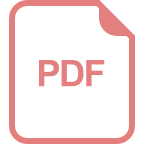
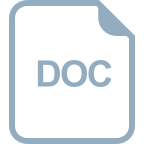
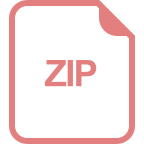
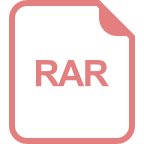
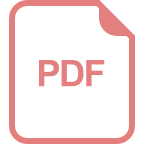
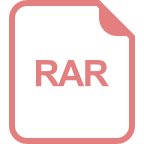
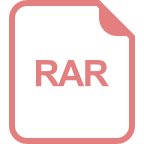
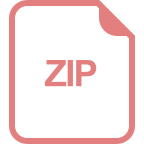
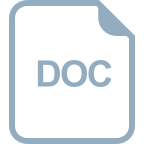
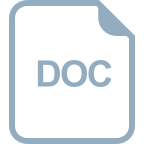
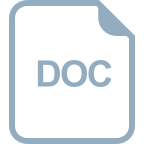
