go 结构体数组排序
时间: 2023-11-24 12:50:26 浏览: 36
以下是对Go语言中结构体数组按照年龄大小从小到大进行排序的例子:
```go
type Student struct {
Name string
Age int
}
type StudentArray []Student
func (s StudentArray) Len() int {
return len(s)
}
func (s StudentArray) Swap(i, j int) {
s[i], s[j] = s[j], s[i]
}
func (s StudentArray) Less(i, j int) bool {
return s[i].Age < s[j].Age
}
func main() {
students := []Student{
{Name: "tom", Age: 10},
{Name: "smith", Age: 12},
{Name: "danny", Age: 13},
{Name: "jack", Age: 12},
{Name: "boom", Age: 12},
}
sort.Sort(StudentArray(students))
fmt.Println(students)
}
```
相关问题
golang 结构体数组 按时间排序
可以使用 Go 语言中的 `sort` 包来对结构体数组按时间排序。具体步骤如下:
1. 定义结构体,包含时间属性和其他属性。
```go
type MyStruct struct {
Time time.Time
// other fields
}
```
2. 定义结构体数组,并初始化。
```go
myStructs := []MyStruct {
{Time: time.Now(), /* other fields */},
{Time: time.Now().Add(-time.Hour), /* other fields */},
// more elements
}
```
3. 定义排序函数,用于比较结构体的时间属性。
```go
func ByTime(s1, s2 *MyStruct) bool {
return s1.Time.Before(s2.Time)
}
```
4. 调用 `sort.Sort()` 函数,传入结构体数组和排序函数。
```go
sort.Slice(myStructs, func(i, j int) bool {
return ByTime(&myStructs[i], &myStructs[j])
})
```
这样,结构体数组 `myStructs` 就会按照时间属性从早到晚排序。
golang 结构体数组 按字符串时间类型排序
可以使用 Go 语言中的 `sort` 包和 `time` 包来对结构体数组按字符串表示的时间排序。具体步骤如下:
1. 定义结构体,包含字符串表示的时间属性和其他属性。
```go
type MyStruct struct {
TimeStr string
// other fields
}
```
2. 定义结构体数组,并初始化。
```go
myStructs := []MyStruct {
{TimeStr: "2021-11-10 10:00:00", /* other fields */},
{TimeStr: "2021-11-11 09:00:00", /* other fields */},
// more elements
}
```
3. 定义排序函数,用于比较结构体的时间属性。
```go
func ByTime(s1, s2 *MyStruct) bool {
t1, _ := time.Parse("2006-01-02 15:04:05", s1.TimeStr)
t2, _ := time.Parse("2006-01-02 15:04:05", s2.TimeStr)
return t1.Before(t2)
}
```
4. 调用 `sort.Sort()` 函数,传入结构体数组和排序函数。
```go
sort.Slice(myStructs, func(i, j int) bool {
return ByTime(&myStructs[i], &myStructs[j])
})
```
这样,结构体数组 `myStructs` 就会按照字符串表示的时间属性从早到晚排序。需要注意的是,排序函数中的时间格式必须和字符串表示的时间格式匹配。在本例中,时间格式为 "2006-01-02 15:04:05",这是 Go 语言中的一个固定时间格式。如果时间格式不匹配,`time.Parse()` 函数将返回错误。
相关推荐
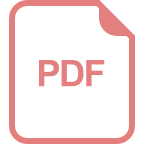
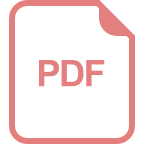
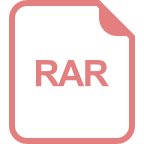
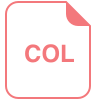
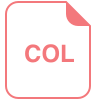
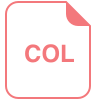
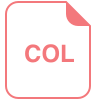







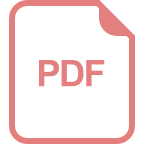