vue 判断indexof
时间: 2023-11-08 20:16:57 浏览: 85
在 Vue 中判断字符串是否包含子字符串可以使用以下方法:
1. 使用 JavaScript 的 `indexOf()` 方法:
```javascript
if (myString.indexOf(subString) !== -1) {
// 包含子字符串
} else {
// 不包含子字符串
}
```
2. 使用 ES6 中的 `includes()` 方法:
```javascript
if (myString.includes(subString)) {
// 包含子字符串
} else {
// 不包含子字符串
}
```
在 Vue 模板中,你可以在条件语句中使用这些判断方法。例如:
```vue
<template>
<div>
<p v-if="myString.includes(subString)">
包含子字符串
</p>
<p v-else>
不包含子字符串
</p>
</div>
</template>
<script>
export default {
data() {
return {
myString: 'Hello World',
subString: 'World'
};
}
}
</script>
```
这样,根据 `myString` 是否包含 `subString` 的结果,将会显示不同的段落内容。
相关问题
vue中indexOf函数
在Vue中,indexOf函数是JavaScript中的一个数组方法,用于返回指定元素在数组中的索引位置。它可以用于判断数组中是否包含某个元素,并返回该元素的索引值。
在Vue中使用indexOf函数的语法如下:
```
array.indexOf(element)
```
其中,array是要进行查找的数组,element是要查找的元素。
如果找到了指定的元素,则返回该元素在数组中的索引值;如果没有找到,则返回-1。
例如,假设有一个数组arr=[1, 2, 3, 4, 5],我们想要查找数字3在数组中的索引位置,可以使用indexOf函数:
```
var index = arr.indexOf(3);
console.log(index); // 输出2
```
这里的index将会输出2,表示数字3在数组arr中的索引位置是2。
需要注意的是,indexOf函数只会返回第一个匹配到的元素的索引值。如果数组中有多个相同的元素,它只会返回第一个匹配到的元素的索引值。
vue2 indexof
引用中提到,在Vue中使用indexOf()报错"Cannot read property 'indexOf' of null"。这个错误通常是因为在使用indexOf()方法之前,没有对数据进行判断或者处理空值的情况。引用中指出,可能是因为在Vue的双向绑定数据类型上出现问题。在示例中,初始值定义为空字符串' ',而后来需求更改为多选筛选,初始值应定义为数组[ ]。如果在切换需求时未对初始值进行相应的更改,就可能引发报错。
引用中提到,可以手动编写模糊查询的事件来解决报错问题。在代码中,使用filter方法对数据进行循环,并使用indexOf()方法对指定的属性值进行查找。但报错的代码中,在循环过程中,可能由于e的值未被正确识别或为空等原因,导致indexOf()方法出现报错。为了解决这个问题,可以在filter方法中添加对e的判断,确保e不为空并且能够正确识别。
综上所述,如果在Vue中使用indexOf()报错"Cannot read property 'indexOf' of null",可以通过检查双向绑定的数据类型是否正确,并在使用indexOf()方法之前对数据进行判断,避免出现空值或未定义的情况。此外,还可以手动编写模糊查询的事件,在filter方法中添加对数据的判断,确保能够正确使用indexOf()方法。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *3* [vue模糊查询使用indexOf()报错Cannot read property ‘indexOf‘ of null](https://blog.csdn.net/cc6_66/article/details/115554960)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *2* [【vue报错解决】TypeError: i.indexOf is not a function](https://blog.csdn.net/FTEzreal/article/details/122875374)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐
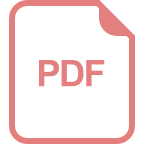
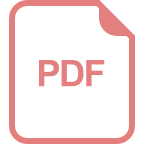
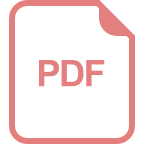












