c语言 只用数组 函数search( ) ——根据姓名用顺序查找方法找出该学生,查找成功返回学生下标,否则返回-1;
时间: 2024-12-24 17:38:55 浏览: 9
在C语言中,如果你想要实现一个只使用数组的功能,比如函数`search()`,它的目的是根据给定的学生姓名在一个已排序的学生数组中找到特定学生的下标。这个函数可能会采用线性查找(顺序查找)的方式,逐个比较每个学生的姓名直到找到匹配项或者遍历完整个数组。下面是一个简单的函数实现示例:
```c
#include <stdio.h>
#include <string.h>
// 定义学生结构体
typedef struct {
char name[50];
int index; // 学生的数组下标
} Student;
// 搜索函数
int search(const Student arr[], int n, const char* target) {
int i;
for (i = 0; i < n; i++) { // 遍历数组
if (strcmp(arr[i].name, target) == 0) { // 如果找到目标名字
return arr[i].index; // 返回对应的学生下标
}
}
return -1; // 没有找到,返回-1
}
// 示例主函数
int main() {
// 假设有一个已经按姓名排序的学生数组
Student students[] = {
{"张三", 0},
{"李四", 1},
{"王五", 2},
// 更多学生...
};
int student_count = sizeof(students) / sizeof(students[0]);
const char* target_name = "李四"; // 要搜索的名字
int result = search(students, student_count, target_name);
if (result != -1) {
printf("找到了学生,下标为:%d\n", result);
} else {
printf("未找到学生。\n");
}
return 0;
}
```
阅读全文
相关推荐




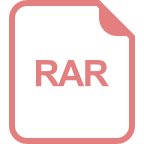








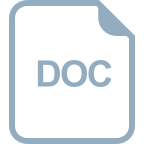
