使用tensorflow创建指定shape的tensor
时间: 2023-09-17 20:09:01 浏览: 64
要使用 TensorFlow 创建指定形状的张量,可以使用 `tf.zeros`、`tf.ones`、`tf.fill` 或者直接使用 Python 的列表或 NumPy 数组来创建。
以下是几种常见的创建张量的方法示例:
1. 使用 `tf.zeros` 创建全零张量:
```python
import tensorflow as tf
shape = (2, 3) # 指定形状为 (2, 3)
zeros_tensor = tf.zeros(shape)
print(zeros_tensor)
```
输出结果:
```
<tf.Tensor: shape=(2, 3), dtype=float32, numpy=
array([[0., 0., 0.],
[0., 0., 0.]], dtype=float32)>
```
2. 使用 `tf.ones` 创建全一张量:
```python
import tensorflow as tf
shape = (2, 3) # 指定形状为 (2, 3)
ones_tensor = tf.ones(shape)
print(ones_tensor)
```
输出结果:
```
<tf.Tensor: shape=(2, 3), dtype=float32, numpy=
array([[1., 1., 1.],
[1., 1., 1.]], dtype=float32)>
```
3. 使用 `tf.fill` 创建指定值的张量:
```python
import tensorflow as tf
shape = (2, 3) # 指定形状为 (2, 3)
value = 5 # 指定值为 5
filled_tensor = tf.fill(shape, value)
print(filled_tensor)
```
输出结果:
```
<tf.Tensor: shape=(2, 3), dtype=int32, numpy=
array([[5, 5, 5],
[5, 5, 5]], dtype=int32)>
```
4. 使用 Python 列表或 NumPy 数组创建张量:
```python
import tensorflow as tf
import numpy as np
list_data = [[1, 2, 3], [4, 5, 6]] # 使用 Python 列表
numpy_data = np.array([[1, 2, 3], [4, 5, 6]]) # 使用 NumPy 数组
list_tensor = tf.convert_to_tensor(list_data)
numpy_tensor = tf.convert_to_tensor(numpy_data)
print(list_tensor)
print(numpy_tensor)
```
输出结果:
```
<tf.Tensor: shape=(2, 3), dtype=int32, numpy=
array([[1, 2, 3],
[4, 5, 6]], dtype=int32)>
<tf.Tensor: shape=(2, 3), dtype=int64, numpy=
array([[1, 2, 3],
[4, 5, 6]])>
```
希望这些示例能帮助你创建指定形状的张量。如果你有任何进一步的问题,请随时提问!
相关推荐
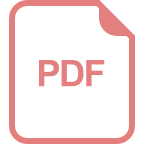
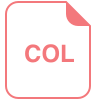
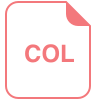
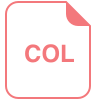
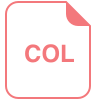
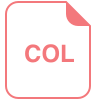
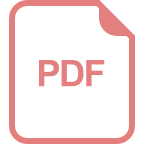
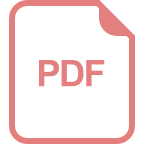
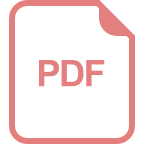
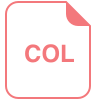
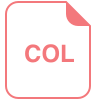
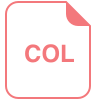
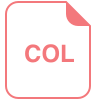
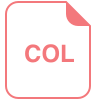
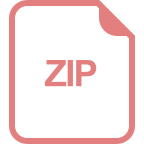