c语言实现,已知两点的距离和经纬度和第三点到第一点的距离和第三点到第二点的距离,求第三点的经纬度
时间: 2024-04-14 10:27:31 浏览: 85
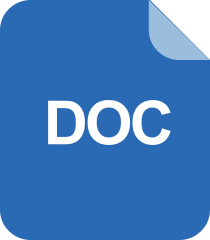
已知两点经纬度组成的直线_求指定距离的第三点经纬度公式

要求解已知两点的距离、经纬度,以及第三点到第一点和第三点到第二点的距离,可以使用三角形定位法进行计算。下面是一个C语言示例代码,用于计算第三点的经纬度:
```c
#include <stdio.h>
#include <math.h>
#define RADIUS 6371 // 地球半径,单位为公里
struct point {
double latitude;
double longitude;
};
double deg2rad(double deg) {
return deg * M_PI / 180;
}
double rad2deg(double rad) {
return rad * 180 / M_PI;
}
struct point calculate_third_point(struct point p1, struct point p2, double distance1, double distance2) {
double lat1 = deg2rad(p1.latitude);
double lon1 = deg2rad(p1.longitude);
double lat2 = deg2rad(p2.latitude);
double lon2 = deg2rad(p2.longitude);
double delta_lon = lon2 - lon1;
double a = pow(sin((lat2 - lat1) / 2), 2) + cos(lat1) * cos(lat2) * pow(sin(delta_lon / 2), 2);
double c = 2 * atan2(sqrt(a), sqrt(1 - a));
double bearing = atan2(sin(delta_lon) * cos(lat2), cos(lat1) * sin(lat2) - sin(lat1) * cos(lat2) * cos(delta_lon));
double angular_distance1 = distance1 / RADIUS;
double angular_distance2 = distance2 / RADIUS;
double third_point_lat = asin(sin(lat1) * cos(angular_distance1) + cos(lat1) * sin(angular_distance1) * cos(bearing));
double third_point_lon = lon1 + atan2(sin(bearing) * sin(angular_distance1) * cos(lat1), cos(angular_distance1) - sin(lat1) * sin(third_point_lat));
struct point third_point;
third_point.latitude = rad2deg(third_point_lat);
third_point.longitude = rad2deg(third_point_lon);
return third_point;
}
int main() {
struct point p1 = {40.7128, -74.0060}; // 已知的第一个点的纬度和经度
struct point p2 = {34.0522, -118.2437}; // 已知的第二个点的纬度和经度
double distance1 = 500; // 第三个点到第一个点的距离,单位为公里
double distance2 = 800; // 第三个点到第二个点的距离,单位为公里
struct point third_point = calculate_third_point(p1, p2, distance1, distance2);
printf("Third point: Latitude - %lf, Longitude - %lf\n", third_point.latitude, third_point.longitude);
return 0;
}
```
这段代码使用了与前面示例相同的球面三角学方法,但在计算第三点经纬度时,使用了两个已知距离。请注意,这只是一个简单的示例代码,实际应用中需要考虑更多因素,如地球的椭球形状、精度误差等。
阅读全文
相关推荐


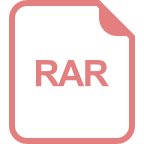
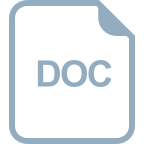


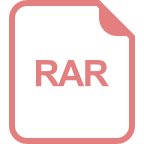
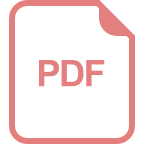
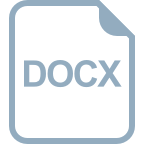






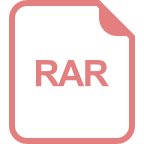