react函数式组件父组件使用ref调用子组件的方法
时间: 2024-08-16 09:06:46 浏览: 32
在React中,函数式组件通常不直接支持通过`ref`访问并调用子组件的方法,因为函数式组件没有传统的生命周期方法和实例状态。但是,你可以通过以下几种方式间接地实现这个目标:
1. **forwardRef** API:`forwardRef`是一个高阶函数,可以让你将`ref`传递给子组件,并从外部接收一个回调,这个回调可以在适当的时候调用子组件的方法。
```jsx
import { forwardRef } from 'react';
function ParentComponent({ childProps }) {
const childRef = React.useRef(null);
const handleClick = () => {
if (childRef.current) {
childRef.current.childMethod();
}
};
return (
<ChildComponent ref={childRef} {...childProps} />
// 在需要的地方添加按钮事件处理,触发 handleClick
<button onClick={handleClick}>Call Child Method</button>
);
}
const ChildComponent = forwardRef((props, ref) => {
// ...
useEffect(() => {
// 当子组件挂载后,设置子方法可被外部引用
ref.current = { childMethod };
}, [ref]);
// ...
});
```
2. **useImperativeHandle**:如果你确定子组件暴露了某个特定的方法,可以使用`useImperativeHandle`来提供一个自定义的API,让父组件能够像操作DOM元素一样调用它。
```jsx
import React, { useRef, useImperativeHandle } from 'react';
function ParentComponent({ childProps }) {
const childRef = useRef(null);
useImperativeHandle(childRef, () => ({
callChildMethod: () => {
childRef.current.callChildMethod();
},
}));
// 使用 ImperativeHandle 的 API
return (
<ChildComponent ref={childRef} {...childProps} />
// ...
<button onClick={() => childRef.current.callChildMethod()}>Call Child Method</button>
);
}
// ...在 ChildComponent 中定义 callChildMethod 方法
```
请注意,在实际应用中,尽量避免过度依赖`ref`直接操作子组件内部的状态或方法,除非有非常明确的需求。通常,更好的做法是利用 props 和 React 的其他特性来管理组件间通信。
相关推荐
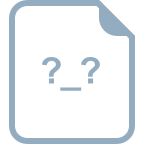
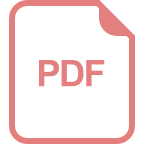
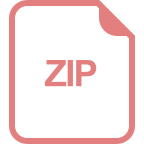














