python滑动验证码识别
时间: 2025-01-04 11:29:30 浏览: 24
### 如何使用Python实现滑动验证码识别
#### 方法一:基于OpenCV的模板匹配方法
为了处理滑动验证码,可以采用图像处理技术来定位滑块的位置以及计算其应该被拖拽的距离。具体来说,可以通过比较两张图片——一张是没有遮挡物的标准背景图,另一张是有遮挡物的目标图——找到两者的差异区域即为缺口所在之处。
```python
import cv2
import numpy as np
def find_slider_position(bg_img_path, target_img_path):
bg_img = cv2.imread(bg_img_path)
target_img = cv2.imread(target_img_path)
# 转换成灰度图并做二值化处理
gray_bg = cv2.cvtColor(bg_img, cv2.COLOR_BGR2GRAY)
_, binary_bg = cv2.threshold(gray_bg, 150, 255, cv2.THRESH_BINARY_INV)
gray_target = cv2.cvtColor(target_img, cv2.COLOR_BGR2GRAY)
_, binary_target = cv2.threshold(gray_target, 150, 255, cv2.THRESH_BINARY_INV)
# 计算两个图像之间的绝对差分
diff = cv2.absdiff(binary_bg, binary_target)
kernel = np.ones((5, 5), dtype=np.uint8)
dilated_diff = cv2.dilate(diff, kernel=kernel, iterations=1)
contours, _ = cv2.findContours(dilated_diff.copy(), cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
if not contours:
raise Exception("无法检测到滑块")
contour = max(contours, key=cv2.contourArea)
(x, y, w, h) = cv2.boundingRect(contour)
return x + int(w / 2)
slider_pos_x = find_slider_position('./bg_img.png', './target_img.png')
print(f"滑块应位于 X 坐标: {slider_pos_x}")
```
此段代码展示了如何利用`cv2`库来进行基本的图像预处理和轮廓查找工作,从而确定滑块应当放置的具体位置[^1]。
#### 方法二:借助OCR服务进行缺口识别
另一种方式是调用第三方API完成更复杂的视觉任务,比如百度AI平台提供的文字识别(OCR)、物体检测等功能也可以用来辅助解决这类问题。下面是一个简单的例子说明怎样发送HTTP请求给特定的服务端点以获得缺口坐标:
```python
import requests
from PIL import Image
import io
def get_gap_location(image_file_path, access_token='your_access_token'):
url = "https://aip.baidubce.com/rest/2.0/solution/v1/img_censor/user_defined"
headers = {
'Content-Type': 'application/x-www-form-urlencoded',
'Authorization': f'Bearer {access_token}'
}
img_data = open(image_file_path, mode="rb").read()
params = {"image": base64.b64encode(img_data).decode()}
response = requests.post(url=url, data=params, headers=headers)
result = response.json()
try:
location_left = result['results'][0]['location']['left']
print(f"缺口左侧边缘距离左边界像素数为{location_left}.")
return location_left
except KeyError as e:
print(e)
print(result)
raise ValueError("未能解析返回的数据")
gap_loc = get_gap_location("./bg_img.png")
```
上述脚本中包含了向百度智能云提交待分析图片的过程,并从中提取出有关缺口的信息[^5]。
---
阅读全文
相关推荐







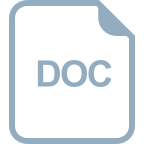

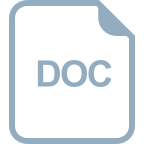
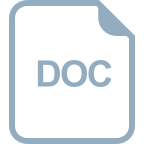
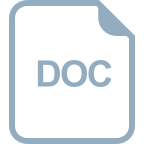
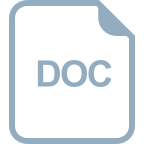



