python爬虫抓取百度贴吧网页评价
时间: 2024-12-26 10:27:26 浏览: 5
### 使用Python编写爬虫程序抓取百度贴吧网页评价
为了实现这一目标,可以采用多线程技术以及正则表达式处理页面内容。具体来说:
通过`requests`库发送HTTP请求并接收响应,利用`BeautifulSoup`解析HTML文档结构以便于定位所需的数据节点[^2]。
对于并发操作,推荐使用`concurrent.futures.ThreadPoolExecutor`来管理多个工作线程执行任务,这相比传统的`threading`模块提供了更简洁高效的接口[^3]。
针对特定模式的信息提取,则依赖于强大的正则表达式引擎——`re`库来进行匹配和筛选有效负载[^4]。
下面是一个简单的代码框架用于说明上述过程:
```python
import requests
from bs4 import BeautifulSoup
import re
from concurrent.futures import ThreadPoolExecutor, as_completed
def fetch_page(url):
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64)',
}
response = requests.get(url, headers=headers)
if response.status_code == 200:
return response.text
else:
raise Exception(f"Failed to load page {url}")
def parse_comments(html_content):
soup = BeautifulSoup(html_content, "html.parser")
comments_section = soup.find_all('div', class_='d_post_content') # 假设评论位于此类名下
pattern = r'[\w\.-]+@[\w\.-]+' # 此处仅为示例,实际应调整为适合捕捉评价文本的规则
extracted_data = []
for comment in comments_section:
matches = re.findall(pattern, str(comment))
cleaned_text = ''.join(matches).strip()
if cleaned_text:
extracted_data.append(cleaned_text)
return extracted_data
base_url = "https://tieba.baidu.com/p/{post_id}?pn={page_number}"
post_id = input("Enter the post ID:")
max_pages = int(input("How many pages do you want to scrape?"))
with ThreadPoolExecutor(max_workers=5) as executor:
futures_to_urls = {}
for i in range(1, max_pages + 1):
url = base_url.format(post_id=post_id, page_number=i)
future = executor.submit(fetch_page, url)
futures_to_urls[future] = url
all_results = []
for future in as_completed(futures_to_urls):
try:
html = future.result()
results = parse_comments(html)
all_results.extend(results)
except Exception as exc:
print('%r generated an exception: %s' % (futures_to_urls[future], exc))
print(all_results[:10]) # 输出前十个结果作为样本展示
```
此脚本展示了如何构建一个多线程环境下的网络爬虫应用程序,它能够有效地遍历指定范围内的帖子页码,并从中抽取感兴趣的字段值。需要注意的是,在真实环境中应当遵循网站的服务条款,并考虑设置合理的延时间隔以免给服务器造成过大压力。
阅读全文
相关推荐
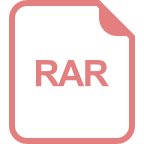
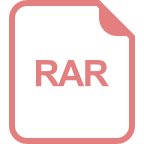
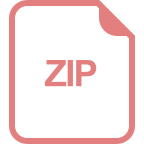
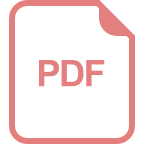
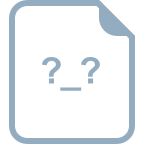
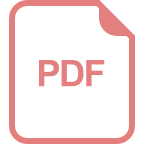
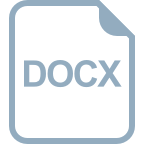
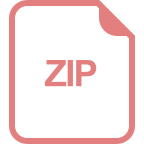
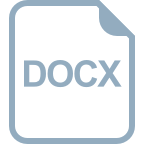
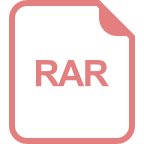
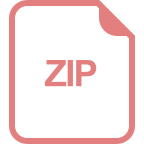
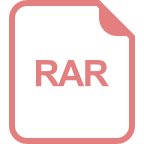
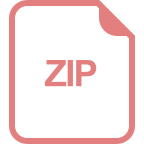
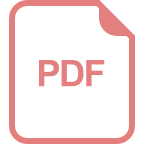
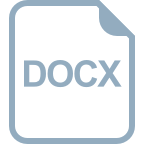
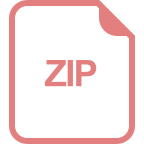