python脚本对xml解析
时间: 2024-08-31 15:01:23 浏览: 121
Python中有多种库可以用于XML解析,其中最常用的是`ElementTree`和第三方库如`lxml`、`xml.etree.ElementTree`(它是标准库的一部分)和`BeautifulSoup`。以下是使用`ElementTree`的一个简单例子:
```python
import xml.etree.ElementTree as ET
# 解析XML文件
tree = ET.parse('example.xml')
root = tree.getroot()
# 遍历元素
for child in root:
print(f"Tag: {child.tag}, Text: {child.text}")
# 提取特定标签的内容
data = root.findall('.//tag_name') # 使用XPath表达式获取tag_name标签的所有内容
for item in data:
print(item.text)
相关问题
用Python根据现有xml脚本和需求文件,生成新xml脚本
好的,生成新xml脚本是一个常见的任务,可以使用Python中的xml库来实现。具体步骤如下:
1. 读取现有的xml脚本,可以使用xml.etree.ElementTree库中的parse函数来解析xml文件并获取根元素。
```python
import xml.etree.ElementTree as ET
tree = ET.parse('现有xml脚本.xml')
root = tree.getroot()
```
2. 根据需求文件中的要求修改现有的xml脚本,可以使用ElementTree库中的find和findall函数来查找对应的元素,并使用set函数来修改元素的属性。
```python
# 查找对应的元素
element = root.find('.//要修改的元素')
# 修改元素的属性
element.set('属性名', '新属性值')
```
3. 创建新的xml元素并添加到根元素中,可以使用SubElement函数来创建新元素,并使用append函数将其添加到根元素中。
```python
# 创建新元素
new_element = ET.SubElement(root, '新元素')
# 设置新元素的属性和文本内容
new_element.set('属性名', '属性值')
new_element.text = '文本内容'
```
4. 将修改后的xml树写入新的xml文件中,可以使用ElementTree库中的ElementTree函数来创建新的xml树,并使用write函数将其写入文件。
```python
# 创建新的xml树
new_tree = ET.ElementTree(root)
# 将xml树写入文件
new_tree.write('新xml脚本.xml', encoding='utf-8', xml_declaration=True)
```
以上就是根据现有xml脚本和需求文件生成新xml脚本的基本步骤,可以根据具体的需求进行修改和扩展。
用Python根据现有xml脚本和需求文件,生成新xml脚本,举例说明
好的,我可以给您举一个例子。
假设现有一个名为 `example.xml` 的 XML 脚本文件,其内容如下:
```xml
<?xml version="1.0"?>
<catalog>
<book id="001">
<author>John Doe</author>
<title>The Book of John</title>
<genre>Fiction</genre>
<price>20.00</price>
</book>
<book id="002">
<author>Jane Doe</author>
<title>The Book of Jane</title>
<genre>Non-Fiction</genre>
<price>15.00</price>
</book>
</catalog>
```
现在,我们需要根据需求文件生成一个新的 XML 脚本文件。需求文件的内容如下:
```text
- 添加一本名为 "The Book of Bob" 的书,作者为 "Bob Brown",类型为 "Fiction",价格为 25.00。
- 删除 id 为 "002" 的书。
```
我们可以使用 Python 中的 `xml.etree.ElementTree` 模块来解析现有的 XML 脚本文件,并根据需求文件生成新的 XML 脚本。下面是示例代码:
```python
import xml.etree.ElementTree as ET
# 解析现有的 XML 脚本文件
tree = ET.parse('example.xml')
root = tree.getroot()
# 根据需求文件添加新的书籍
new_book = ET.SubElement(root, 'book', {'id': '003'})
new_book_author = ET.SubElement(new_book, 'author')
new_book_author.text = 'Bob Brown'
new_book_title = ET.SubElement(new_book, 'title')
new_book_title.text = 'The Book of Bob'
new_book_genre = ET.SubElement(new_book, 'genre')
new_book_genre.text = 'Fiction'
new_book_price = ET.SubElement(new_book, 'price')
new_book_price.text = '25.00'
# 根据需求文件删除指定的书籍
for book in root.findall('book'):
if book.get('id') == '002':
root.remove(book)
# 生成新的 XML 脚本文件
tree.write('new_example.xml', encoding='utf-8', xml_declaration=True)
```
在上面的示例代码中,我们首先使用 `ET.parse()` 函数解析现有的 XML 脚本文件,然后使用 `ET.SubElement()` 函数根据需求文件添加新的书籍。接着,我们使用 `root.findall()` 函数查找要删除的书籍,并使用 `root.remove()` 函数删除它们。最后,我们使用 `tree.write()` 函数将生成的新的 XML 脚本文件写入到磁盘上。
阅读全文
相关推荐
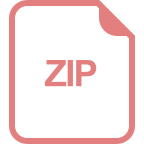
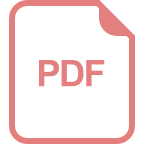
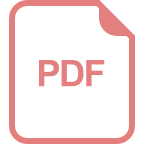
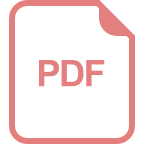
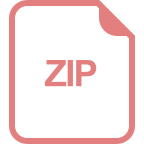
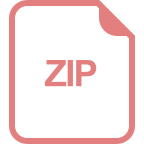
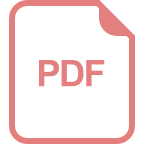
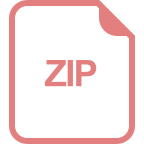
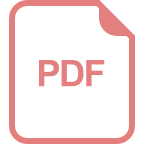
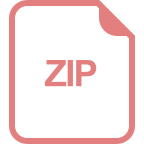
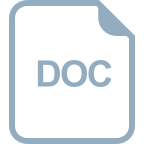
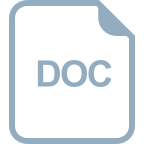
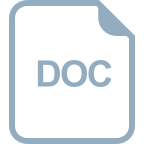
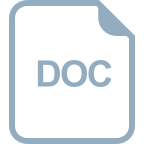
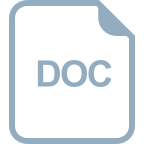
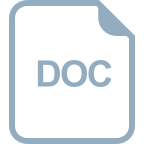
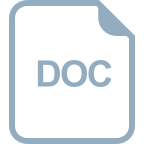