c++中开三次方函数
时间: 2024-11-14 18:13:19 浏览: 14
在 C++ 中,标准库并没有直接提供开立方根的函数,但你可以通过数学库 `cmath` 或者第三方库如 `<complex>` 来计算实数或复数的立方根。如果你只需要处理实数,可以使用 `pow()` 函数配合 `std::sqrt()` 来间接得到:
```cpp
#include <iostream>
#include <cmath>
double cube_root(double x) {
return std::cbrt(x);
}
int main() {
double num = 8.0;
double result = cube_root(num);
std::cout << "The cube root of " << num << " is " << result << std::endl;
return 0;
}
```
如果你需要处理复数,比如 `std::complex` 类型,可以使用其提供的 `abs()` 和 `pow(1./3., complex)` 方法来求解:
```cpp
#include <iostream>
#include <complex>
int main() {
std::complex<double> complex_num{4.0, 2.0 * I}; // I 表示虚数单位
auto abs_value = std::abs(complex_num); // 先求模
auto root = std::pow(abs_value, std::complex<double>(1./3., 0)); // 取立方根
std::cout << "The cube root of (" << complex_num.real() << ", " << complex_num.imag() << ") is (" << root.real() << ", " << root.imag() << ")" << std::endl;
return 0;
}
```
阅读全文
相关推荐
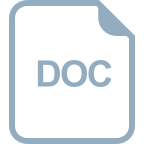
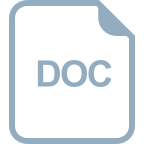
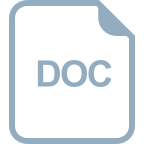
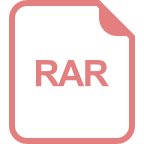
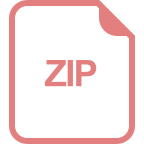
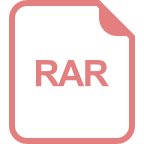
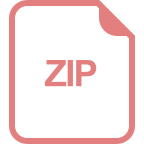
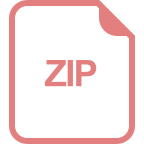
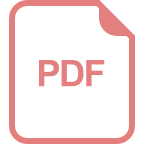
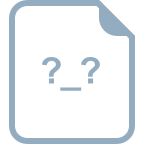
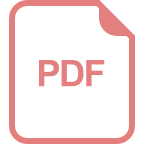
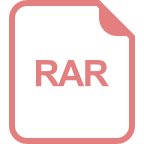
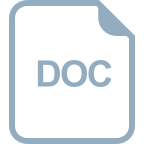