Python爬取资产负债表
时间: 2024-06-10 08:03:27 浏览: 286
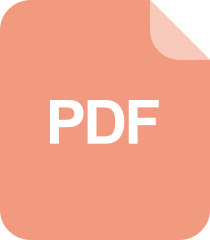
Python爬取豆瓣视频信息代码实例
在Python中,爬取企业的资产负债表通常涉及到网络抓取和数据解析技术,特别是使用像`requests`库来获取网页内容,然后用`BeautifulSoup`或`pandas`等库来处理HTML或XML数据。以下是一个简单的步骤概述:
1. **安装必要的库**:
首先,确保已安装`requests`, `beautifulsoup4`, `lxml`(BeautifulSoup的一个优化版本)以及可能用于解析特定格式(如CSV或XML)的库,如`pandas`。
```bash
pip install requests beautifulsoup4 lxml pandas
```
2. **获取网页内容**:
使用`requests.get()`获取包含资产负债表的网页源代码。假设你要爬取的是某个公司的HTML页面,比如`https://www.example.com/financials`。
```python
import requests
url = 'https://www.example.com/financials'
response = requests.get(url)
html_content = response.text
```
3. **解析HTML**:
使用BeautifulSoup解析HTML内容,找到包含资产负债表数据的部分。这通常涉及到选择器的选择。
```python
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'lxml')
table = soup.find('table', {'class': 'financials-table'}) # 这里的选择器根据实际网页结构变化
```
4. **提取数据**:
识别表中的数据项,如列标题和单元格内容。这可能需要遍历表格元素并进一步解析。
```python
headers = [header.text for header in table.find_all('th')]
data_rows = table.find_all('tr')
# 假设每一行的数据在td标签中
data = [[cell.text for cell in row.find_all('td')] for row in data_rows]
```
5. **存储数据**:
最后,你可以将数据存储到CSV、Excel或者直接转化为pandas DataFrame,便于后续分析。
```python
import pandas as pd
df = pd.DataFrame(data, columns=headers)
df.to_csv('balance_sheet.csv', index=False)
```
阅读全文
相关推荐
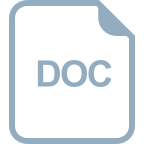
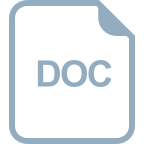
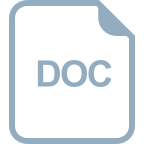






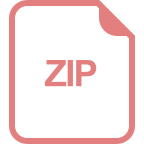
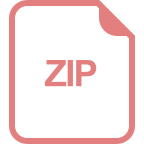
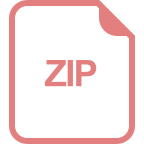
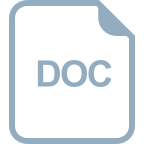
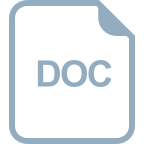
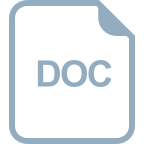
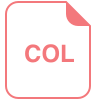
