写一个java模拟购物网站购物车的代码
时间: 2024-12-06 09:22:04 浏览: 46
在Java中,我们可以创建一个简单的模拟购物车(Shopping Cart)的类,它通常包含商品列表(List<CartItem>),以及添加、删除和查看商品的方法。这里是一个简化的例子:
```java
import java.util.ArrayList;
import java.util.List;
class CartItem {
String productName;
double price;
int quantity;
public CartItem(String productName, double price) {
this.productName = productName;
this.price = price;
this.quantity = 1;
}
// getters and setters for the properties
public void setQuantity(int quantity) {
this.quantity = quantity;
}
public double getTotal() {
return price * quantity;
}
}
public class ShoppingCart {
private List<CartItem> items;
public ShoppingCart() {
this.items = new ArrayList<>();
}
// 添加商品到购物车
public void addItem(CartItem item) {
items.add(item);
}
// 删除购物车中的特定商品
public boolean removeItem(String productName) {
for (CartItem item : items) {
if (item.productName.equals(productName)) {
items.remove(item);
return true; // 成功移除
}
}
return false; // 未找到该商品
}
// 查看购物车总价
public double getTotalCost() {
double total = 0;
for (CartItem item : items) {
total += item.getTotal();
}
return total;
}
// 打印购物车内容
public void printCart() {
for (CartItem item : items) {
System.out.println("产品:" + item.productName + ", 数量:" + item.quantity + ", 总价:" + item.getTotal());
}
}
}
```
在这个例子中,`CartItem`类代表购物车中的每个商品,`ShoppingCart`类管理商品列表。你可以通过`addItem`方法添加商品,`removeItem`方法移除商品,`getTotalCost`计算总价,`printCart`方法显示购物车内所有商品的信息。
阅读全文
相关推荐







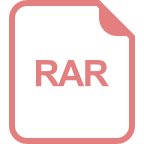




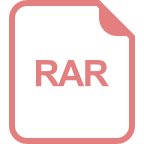





