栈的表达式求值,用C/C++语言编码实现。 1、能对简单的表达式进行求值。 2、能对含有浮点数以及含括号的表达式进行求值。 3、代码格式规范、对异常有处理。
时间: 2024-10-29 22:24:18 浏览: 28
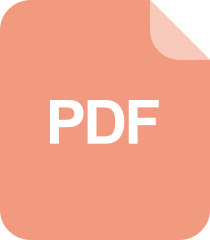
浅谈C/C++ 语言中的表达式求值
栈的表达式求值通常涉及到逆波兰表示法(Reverse Polish Notation,RPN),也被称为后缀表达式,因为操作符位于它们所作用的操作数之后。在C/C++中实现这样的功能可以分为以下几个步骤:
1. **解析输入表达式**:
先将输入的中缀表达式转换成后缀表达式,这个过程可能需要使用到括号优先算法。
```cpp
#include <stack>
#include <string>
std::string infixToPostfix(const std::string &infix) {
std::stack<char> opStack;
std::string postfix;
for (char c : infix) {
if (isalnum(c)) // 数字或变量
postfix += c;
else if (c == '(') { // 左括号压栈
opStack.push(c);
} else if (c == ')') { // 右括号出栈并处理嵌套
while (!opStack.empty() && opStack.top() != '(')
postfix += opStack.pop();
opStack.pop(); // 出栈左括号
} else { // 操作符
while (!opStack.empty() && precedence(c) <= precedence(opStack.top()))
postfix += opStack.pop();
opStack.push(c);
}
}
while (!opStack.empty())
postfix += opStack.pop();
return postfix;
}
```
2. **计算后缀表达式**:
创建两个栈,一个用于存储操作数,另一个用于存储操作符。遍历后缀表达式,当遇到数字就入栈,遇到操作符则比较它与栈顶操作符的优先级,若当前操作符优先级更高,则弹出所有比它优先级低的操作符,直到找到合适的位置。
```cpp
int evaluatePostfix(std::string postfix) {
std::stack<int> numStack;
for (char c : postfix) {
if (isdigit(c))
numStack.push(c - '0');
else {
int right = numStack.top(); numStack.pop();
int left = numStack.top(); numStack.pop();
numStack.push(evalOp(c, left, right));
}
}
return numStack.top();
}
// 假设我们已经有了evalOp函数来计算二元操作,如加法、减法等
int evalOp(char op, int a, int b) {
switch (op) {
case '+': return a + b;
case '-': return a - b;
// 添加更多其他运算符
}
}
```
3. **异常处理**:
需要检查输入的有效性和堆栈溢出等情况。例如,如果操作符数少于预期的运算数,或者遇到无效字符,应抛出错误。
```cpp
try {
std::string postfix = infixToPostfix(expression); // 转换表达式
int result = evaluatePostfix(postfix); // 计算结果
// 输出或返回结果
} catch (const std::exception &e) {
std::cerr << "Error: " << e.what() << '\n';
}
```
以上是一个基本的栈实现表达式求值的例子,实际应用中可能还需要处理更复杂的情况,比如浮点数的支持。注意,上述代码没有包括完整的异常处理和所有必要的错误检查,你需要根据需求进一步完善。
阅读全文
相关推荐
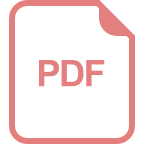
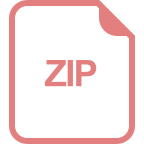
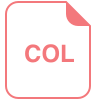
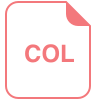
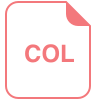
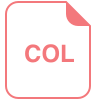
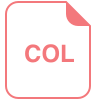
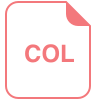
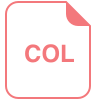

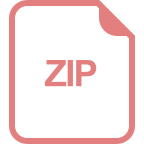