如何在Java中实现在Windows Form上显示一个彩色滚动的字符串'我正在欣赏+歌名'?每字符的颜色应不同,并且每隔一秒钟自动变换颜色,字符串应该从右往左滚动,请提供详细的代码实现步骤。
时间: 2025-01-05 08:46:36 浏览: 6
在Java中,特别是在Windows Forms应用中实现彩色滚动字符串的功能通常需要使用`System.Windows.Forms.Timer`配合`ConsoleColor`类来动态改变颜色。下面是一个简化的示例,展示如何在一个控制台窗口中实现这个功能,尽管这不是原生的Windows Form,但在.NET框架下可以作为一个起点:
```java
import java.awt.Color;
import java.util.Random;
import javax.swing.JFrame;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
import javax.swing.Timer;
public class ColorfulScrollString {
private JFrame frame;
private JTextArea textArea;
private Timer timer;
private String message = "我正在欣赏+歌名";
private Random random = new Random();
private int currentIndex = message.length() - 1;
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
try {
ColorfulScrollString window = new ColorfulScrollString();
window.frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
public ColorfulScrollString() {
// 创建一个新的Windows Form
frame = new JFrame("彩色滚动字符串");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// 设置文本区,用于显示滚动字符串
textArea = new JTextArea(10, 50); // 高度和宽度自行调整
JScrollPane scrollPane = new JScrollPane(textArea);
frame.add(scrollPane);
// 每秒滚动一次
timer = new Timer(1000, new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
if (currentIndex >= 0) {
textArea.setForeground(frame.getBackground()); // 清除当前颜色
textArea.append(message.charAt(currentIndex--)); // 右向滚动并添加字符
colorize(); // 更新下一个字符的颜色
} else {
textArea.setText(""); // 当滚动到最左边时,清空文本区
currentIndex = message.length() - 1; // 从右边开始再循环
}
}
});
timer.start();
}
private void colorize() {
textArea.setForeground(Color.argb(128, random.nextInt(256), random.nextInt(256), random.nextInt(256))); // 随机生成RGB值
}
}
```
注意这只是一个基础的示例,实际应用中可能需要对滚动速度、颜色范围等进行更精细的设置。此外,由于JFrame在Windows Forms中更为常用,上述代码适用于Swing而不是原生的Windows Forms。
阅读全文
相关推荐












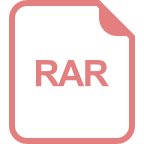


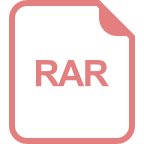
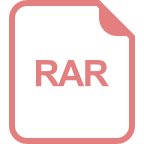

