C++生成三个利用goto语句的代码题
时间: 2024-12-11 09:44:39 浏览: 13
在C++中,虽然现代编程推荐使用控制结构如if-else、循环等,但有时出于教学目的或者特定的需求,我们可能会遇到需要使用`goto`语句的情况。下面是一个简单的例子,展示了如何使用`goto`来实现三种基本功能:
1. **跳转到标记位置**: 这是一种常见的goto使用场景,假设我们要在循环内进行条件判断并退出。
```cpp
int main() {
int num = 0;
for (;;) { // infinite loop
if (num > 5) {
goto exit_loop; // jump to the marked point
}
cout << "Number is: " << num << endl;
num++;
}
exit_loop:
cout << "Loop exited with number: " << num << endl;
return 0;
}
```
2. **分支流程控制**: 可以通过`goto`从一个函数内部直接跳转到另一个函数。
```cpp
void functionA() {
cout << "In Function A" << endl;
goto functionB; // jump to Function B
}
void functionB() {
cout << "In Function B" << endl;
}
```
3. **异常处理**: 虽然这不是最佳实践,但在某些情况下,`goto`可以用于简单地结束异常处理流程。
```cpp
try {
// Some code that may throw an exception
} catch (const std::exception& e) {
cerr << "Caught exception: " << e.what() << endl;
goto cleanup; // jump to clean-up section
}
// Normal flow continues here
cleanup:
cout << "Cleaning up..." << endl;
```
请注意,尽管上述代码展示了`goto`的用法,但现代C++编程通常更倾向于使用`switch`、`while`、`do-while`、`break`和`continue`等控制结构,因为它们提供了更好的可读性和维护性。
阅读全文
相关推荐










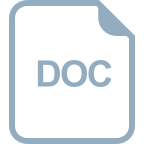

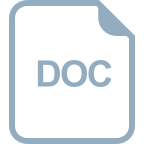





