java反射判断对象字段类型
时间: 2024-08-27 15:02:16 浏览: 60
在Java中,反射API允许我们在运行时检查和操作对象的信息,包括其字段类型。你可以使用`java.lang.reflect.Field`类及其相关方法来实现这个功能。下面是一个简单的示例:
```java
import java.lang.reflect.Field;
public class Main {
public static void main(String[] args) throws NoSuchFieldException, IllegalAccessException {
// 假设有一个名为Person的对象,它有name和age两个字段
Person person = new Person("Alice", 25);
// 获取Person类的Class对象
Class<?> clazz = person.getClass();
// 使用getDeclaredField获取name和age字段
Field nameField = clazz.getDeclaredField("name");
Field ageField = clazz.getDeclaredField("age");
// 检查并打印字段类型
System.out.println("Name field type: " + nameField.getType().getSimpleName());
System.out.println("Age field type: " + ageField.getType().getSimpleName());
// 设置Accessible为true,因为默认情况下,私有字段是不可见的
nameField.setAccessible(true);
ageField.setAccessible(true);
// 如果字段是字符串类型,尝试转换为Integer类型(假设存在类型不匹配的情况)
if (nameField.getType() == String.class) {
try {
int ageAsInt = Integer.parseInt(nameField.get(person).toString());
System.out.println("Converted age as Integer: " + ageAsInt);
} catch (NumberFormatException e) {
System.out.println("Invalid age format");
}
}
}
}
```
在这个例子中,我们首先获取了`person`对象所属的类的`Class`对象,然后通过`getField`方法找到对应的字段,最后使用`getType()`获取字段的类型。
阅读全文
相关推荐
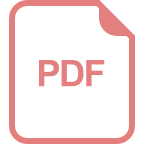
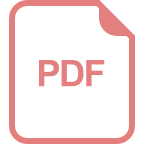
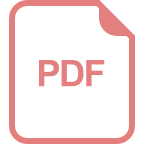






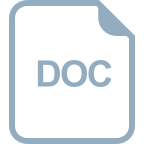
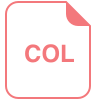





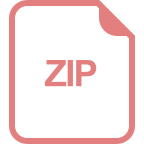
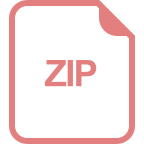
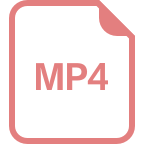