如何使用Pygame库实现Python贪吃蛇?
时间: 2024-05-13 20:11:58 浏览: 19
使用 Pygame 库实现贪吃蛇游戏主要分为以下几个步骤:
1. 导入 Pygame 库并初始化 Pygame 环境。
```python
import pygame
pygame.init()
```
2. 设置游戏窗口和游戏界面。
```python
# 设置游戏窗口大小
WINDOW_WIDTH = 600
WINDOW_HEIGHT = 400
# 设置游戏背景颜色
BG_COLOR = (255, 255, 255)
# 初始化游戏窗口
screen = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
# 设置游戏窗口标题
pygame.display.set_caption('Python贪吃蛇')
```
3. 定义蛇的初始位置和移动方向,以及食物的位置。
```python
# 定义蛇的初始位置和移动方向
snake_position = [100, 100]
snake_body = [[100, 100], [90, 100], [80, 100]]
snake_direction = 'RIGHT'
# 定义食物的初始位置
food_position = [300, 200]
```
4. 编写游戏主循环,处理用户输入,更新蛇的位置和状态,检测游戏是否结束。
```python
while True:
# 处理用户输入
for event in pygame.event.get():
if event.type == pygame.QUIT:
# 用户点击了关闭按钮,退出游戏
pygame.quit()
sys.exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
# 用户按下了左箭头键,改变蛇的移动方向
snake_direction = 'LEFT'
elif event.key == pygame.K_RIGHT:
# 用户按下了右箭头键,改变蛇的移动方向
snake_direction = 'RIGHT'
elif event.key == pygame.K_UP:
# 用户按下了上箭头键,改变蛇的移动方向
snake_direction = 'UP'
elif event.key == pygame.K_DOWN:
# 用户按下了下箭头键,改变蛇的移动方向
snake_direction = 'DOWN'
# 更新蛇的位置和状态
if snake_direction == 'RIGHT':
snake_position += 10
elif snake_direction == 'LEFT':
snake_position -= 10
elif snake_direction == 'UP':
snake_position -= 10
elif snake_direction == 'DOWN':
snake_position += 10
snake_body.insert(0, list(snake_position))
if snake_position == food_position:
# 蛇吃到了食物,重新生成一个食物位置,并将蛇身体长度加1
food_position = [random.randrange(1, (WINDOW_WIDTH // 10)) * 10,
random.randrange(1, (WINDOW_HEIGHT // 10)) * 10]
else:
# 蛇没有吃到食物,将蛇身体最后一节去掉
snake_body.pop()
# 检测游戏是否结束(蛇是否碰到墙壁或自身)
if snake_position < 0 or snake_position > WINDOW_WIDTH - 10:
game_over()
elif snake_position < 0 or snake_position > WINDOW_HEIGHT - 10:
game_over()
for block in snake_body[1:]:
if snake_position == block:
game_over()
# 绘制游戏界面
screen.fill(BG_COLOR)
for position in snake_body:
pygame.draw.rect(screen, (0, 255, 0), pygame.Rect(
position, position, 10, 10))
pygame.draw.rect(screen, (255, 0, 0), pygame.Rect(
food_position, food_position, 10, 10))
pygame.display.update()
```
5. 编写游戏结束函数和启动函数。
```python
def game_over():
# 游戏结束,显示分数并退出游戏
font = pygame.font.SysFont(None, 50)
text = font.render('Score: ' + str(len(snake_body) - 3), True, (255, 0, 0))
screen.blit(text, (WINDOW_WIDTH // 2 - text.get_width() // 2,
WINDOW_HEIGHT // 2 - text.get_height() // 2))
pygame.display.update()
time.sleep(2)
pygame.quit()
sys.exit()
def main():
# 游戏启动函数
# ...
```
相关推荐
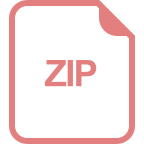
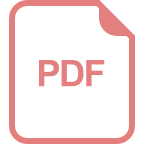
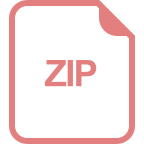














