请帮我写一个java开发的贪吃蛇游戏代码
时间: 2024-09-28 20:06:28 浏览: 35
创建一个简单的Java版贪吃蛇游戏需要一些基础的图形用户界面(GUI)编程,以及对游戏循环、键盘事件处理和数组操作的理解。以下是一个非常基础的示例,使用Swing库进行GUI设计:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.KeyEvent;
import java.util.Random;
public class SnakeGame extends JFrame {
private static final int BOARD_WIDTH = 20;
private static final int BOARD_HEIGHT = 20;
private static final int SNAKE_SPEED = 5;
private final Color[][] board = new Color[BOARD_HEIGHT][BOARD_WIDTH];
private final Snake snake = new Snake();
private boolean gameRunning = true;
public SnakeGame() {
setSize(BOARD_WIDTH * 20, BOARD_HEIGHT * 20);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
initBoard();
initSnake();
addKeyListener(new KeyAdapter() {
@Override
public void keyPressed(KeyEvent e) {
if (gameRunning && e.getKeyCode() == KeyEvent.VK_UP) {
snake.move(Direction.NORTH);
} else if (e.getKeyCode() == KeyEvent.VK_DOWN) {
snake.move(Direction.SOUTH);
} else if (e.getKeyCode() == KeyEvent.VK_LEFT) {
snake.move(Direction.WEST);
} else if (e.getKeyCode() == KeyEvent.VK_RIGHT) {
snake.move(Direction.EAST);
}
}
});
setVisible(true);
}
// 初始化棋盘和蛇
private void initBoard() {
for (int i = 0; i < BOARD_HEIGHT; i++) {
for (int j = 0; j < BOARD_WIDTH; j++) {
board[i][j] = Color.WHITE;
}
}
}
private void initSnake() {
Random random = new Random();
int x = random.nextInt(BOARD_WIDTH);
int y = random.nextInt(BOARD_HEIGHT);
snake.addPoint(x, y);
}
// 游戏逻辑,这里只是一个基本框架,你需要添加碰撞检测和得分计算等
private void gameLoop() {
while (gameRunning) {
// 更新蛇的位置
snake.updatePosition();
// 检查是否吃到食物或撞墙
if (!checkCollision()) {
continue;
}
// 判断游戏结束,关闭窗口并重新初始化
resetGame();
}
}
// 检测碰撞,如果蛇头撞到自己或边界就返回true
private boolean checkCollision() {
Point head = snake.getHead();
return snake.isCollidingWithSelf(head) || !isWithinBoard(head);
}
// 清除屏幕并在随机位置生成新的食物
private void resetGame() {
clearBoard();
initBoard();
int newX = random.nextInt(BOARD_WIDTH - 2) + 1; // 避免蛇头位于边界
int newY = random.nextInt(BOARD_HEIGHT - 2) + 1;
snake.eat(newX, newY);
}
// 其他辅助方法...
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> new SnakeGame());
}
private static boolean isWithinBoard(Point point) {
return point.x >= 0 && point.x < BOARD_WIDTH && point.y >= 0 && point.y < BOARD_HEIGHT;
}
// Snake类和Direction枚举等...
}
// 注意:为了简洁起见,以上代码省略了完整的 Snake 类定义,它应该包含一个方向枚举、头部和身体点列表,以及移动、更新位置和检查碰撞的方法。同时,还需要更复杂的逻辑来处理游戏胜利、失败和食物生成。
```
阅读全文
相关推荐
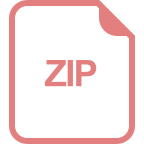
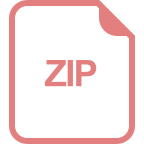
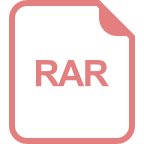
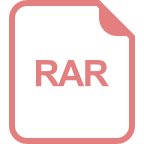
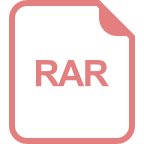
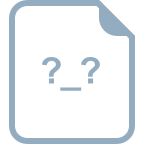
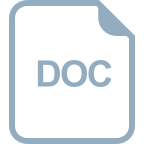
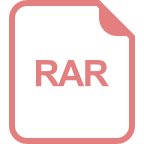
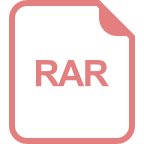
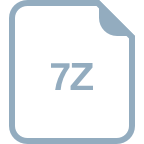
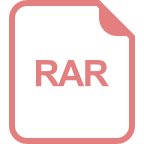
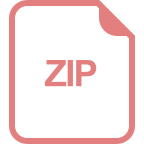
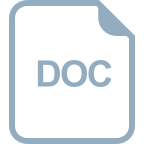
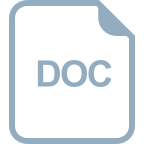
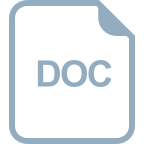
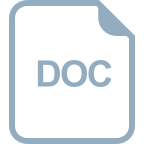
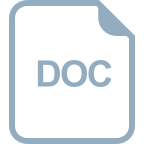
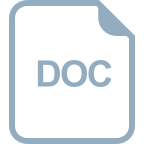
