用c语言编程阶梯电价问题
时间: 2024-11-15 15:31:18 浏览: 52
阶梯电价是一种电费计费方式,根据用户的用电量分段计算费用,通常分为几个档次,比如第一档低价、第二档中价、第三档高价等。在C语言中,你可以使用结构体和条件语句(如if-else或switch)来模拟这样的计费逻辑。
以下是一个简单的阶梯电价示例,假设我们有三个阶梯(0-150度为低价,151-300度为中价,超过300度为高价),每度电价格分别为1元、1.5元和2元:
```c
#include <stdio.h>
// 定义电价结构体
typedef struct {
float lower_threshold;
float upper_threshold;
float price_per_kwh;
} Tariff;
// 定义用户电量和总费用
float user_consumption;
float total_cost = 0;
// 阶梯电价函数
void calculate_bill(Tariff tariffs[], int num_tiers) {
for (int i = 0; i < num_tiers - 1; i++) {
if (user_consumption >= tariffs[i].lower_threshold &&
user_consumption <= tariffs[i].upper_threshold) {
total_cost += user_consumption * tariffs[i].price_per_kwh;
break;
}
}
// 如果超出最后一个阈值,直接按最高价计算
if (user_consumption > tariffs[num_tiers - 1].upper_threshold)
total_cost += (user_consumption - tariffs[num_tiers - 1].upper_threshold) * tariffs[num_tiers - 1].price_per_kwh;
}
int main() {
// 假设阶梯电价数据
Tariff tariffs[] = { {0, 150, 1}, {151, 300, 1.5}, {301, 9999, 2} };
int tiers = sizeof(tariffs) / sizeof(tariffs[0]);
printf("请输入用户电量(单位:度):");
scanf("%f", &user_consumption);
calculate_bill(tariffs, tiers);
printf("用户应付电费:%.2f元\n", total_cost);
return 0;
}
```
在这个程序中,用户需要输入他们的用电量,然后`calculate_bill`函数会根据阶梯电价表计算总费用。
阅读全文
相关推荐
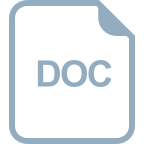
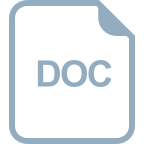
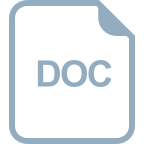
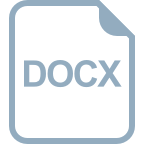
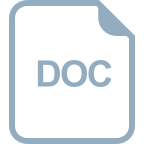
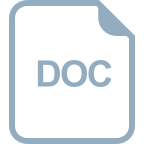
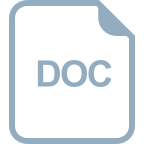
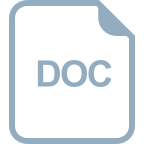
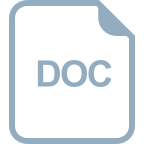


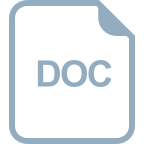
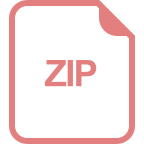
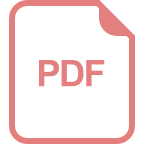
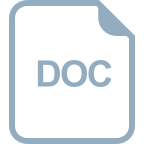
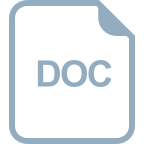
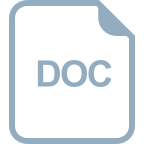
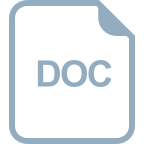