利用指针+函数寻找数组中的最大值及其下标
时间: 2024-10-21 22:02:00 浏览: 28
利用指针和函数来找出数组中的最大值及其下标,通常涉及以下几个步骤:
1. 定义一个函数:这个函数接受一个指向数组首元素的指针以及数组的长度作为参数。例如,在C++中可以这样定义:
```cpp
int findMax(int* arr, int size) {
int max = arr[0]; // 初始化最大值为第一个元素
int maxIndex = 0; // 初始化最大值下标
// 遍历数组
for (int i = 1; i < size; ++i) {
if (arr[i] > max) { // 如果当前元素大于已知的最大值
max = arr[i]; // 更新最大值
maxIndex = i; // 更新最大值下标
}
}
return maxIndex;
}
```
2. 调用函数:传递实际的数组地址和大小给这个函数,它会返回最大值的下标:
```cpp
int array[] = {5, 9, 1, 7, 3}; // 示例数组
int arraySize = sizeof(array) / sizeof(array[0]);
int maxIndex = findMax(array, arraySize);
```
在这个例子中,`maxIndex` 将包含数组中的最大值 `9` 的位置。
相关问题
指针+函数寻找数组中的最大值及其下标
以下是一个使用指针和函数来寻找数组中的最大值及其下标的 C 语言程序:
```c
#include <stdio.h>
void find_max(int arr[], int size, int *max, int *max_index) {
*max = arr[0];
*max_index = 0;
for(int i = 1; i < size; i++) {
if(arr[i] > *max) {
*max = arr[i];
*max_index = i;
}
}
}
int main() {
int arr[] = {1, 3, 5, 7, 9, 8, 6, 4, 2};
int size = sizeof(arr) / sizeof(arr[0]);
int max, max_index;
find_max(arr, size, &max, &max_index);
printf("The maximum value in the array is %d at index %d\n", max, max_index);
return 0;
}
```
该程序定义了一个名为 `find_max` 的函数,它接收一个整数数组 `arr`、数组大小 `size`,以及两个指针,一个指向最大值,另一个指向最大值的下标。函数通过遍历整个数组来找到最大值及其下标,每次比较当前值与之前的最大值,如果当前值更大,就更新最大值及其下标。最后,将最大值和最大值下标通过指针返回给调用者。
程序的 `main` 函数定义了一个整数数组 `arr`,并将其大小计算为 `size`。然后调用 `find_max` 函数来寻找数组中的最大值及其下标,并将它们存储在 `max` 和 `max_index` 变量中。最后,程序输出最大值及其下标。
编写子函数,求出实型数组中的最大值及其下标,在主函数中输出结果。要求:利用指针实现。
下面是一个示例代码,其中 `getMaxIndex` 函数用于求出实型数组中的最大值及其下标,并通过指针修改 `maxValue` 和 `maxIndex` 的值:
```c
#include <stdio.h>
void getMaxIndex(double arr[], int len, double *maxValue, int *maxIndex) {
*maxValue = arr[0];
*maxIndex = 0;
for (int i = 1; i < len; i++) {
if (arr[i] > *maxValue) {
*maxValue = arr[i];
*maxIndex = i;
}
}
}
int main() {
double arr[] = {1.2, -3.4, 5.6, 0.0, 7.8};
int len = sizeof(arr) / sizeof(arr[0]);
double maxValue;
int maxIndex;
getMaxIndex(arr, len, &maxValue, &maxIndex);
printf("Max value: %f\n", maxValue);
printf("Max index: %d\n", maxIndex);
return 0;
}
```
输出结果为:
```
Max value: 7.800000
Max index: 4
```
阅读全文
相关推荐
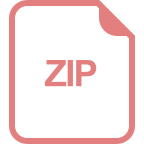
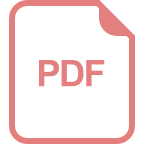













