如何将c链表的内容赋值给c++链表
时间: 2024-06-17 13:00:22 浏览: 154
将C链表的内容赋值给C++链表,需要先将C链表中的元素一个一个复制到C++链表中。这可以通过使用C++中的循环语句和指针来实现。下面是具体的步骤:
1. 创建一个新的C++链表对象。
2. 从C链表的头节点开始,使用循环语句遍历整个C链表。
3. 在每次遍历时,将当前C链表节点的数据复制到一个新的C++链表节点中。
4. 将新的C++节点插入到C++链表中,以便在下次循环时继续添加节点。
5. 当C链表遍历完成后,新的C++链表将包含与原始C链表相同的数据。
以下是参考代码:
```c++
// C代码定义的单向链表节点
typedef struct node {
int data;
struct node* next;
} Node;
// C++代码定义的单向链表节点
class Node {
public:
int data;
Node* next;
Node(int d) {
data = d;
next = NULL;
}
};
// 将C链表赋值给C++链表
void copyList(Node* c_head, Node*& cpp_head) {
// 创建一个新的C++链表对象
cpp_head = NULL;
Node* tail = NULL;
// 遍历整个C链表
while (c_head != NULL) {
// 复制当前C链表节点的数据到新的C++节点中
Node* cpp_node = new Node(c_head->data);
// 插入新的C++节点到链表中
if (cpp_head == NULL) {
cpp_head = cpp_node;
tail = cpp_node;
} else {
tail->next = cpp_node;
tail = tail->next;
}
// 移动到下一个C链表节点
c_head = c_head->next;
}
}
```
阅读全文
相关推荐
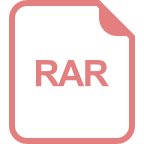



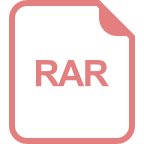










