C++编程实现顺序表,要求顺序表的定义至少包含(1)创建顺序表(2)顺序表的按位查找(3)顺序表的按位插入和按位删除(4)按位获取某个元素等功能;然后实现两个顺序表的并操作(不允许重复元素)和两个有序顺序表的合并操作(允许重复元素)。不使用函数
时间: 2024-10-05 08:04:05 浏览: 34
在C++中,我们可以首先定义一个简单的顺序表结构体,包含数据数组和一些基本操作的函数。这里是一个基础的顺序表实现:
```cpp
#include <iostream>
using namespace std;
// 定义顺序表结构体
struct SeqList {
int* data; // 数据数组
int size; // 当前元素个数
int capacity; // 数组容量
// 创建顺序表,初始为空
SeqList() : data(nullptr), size(0), capacity(5) {}
// 初始化顺序表,分配足够空间
void init(int n) {
if (n > capacity) {
data = new int[n];
capacity = n;
} else {
cout << "Capacity is sufficient." << endl;
}
}
// 按位查找元素
bool search(int key) const {
for (int i = 0; i < size; ++i) {
if (data[i] == key)
return true;
}
return false;
}
// 按位插入元素
void insertAt(int pos, int value) {
if (pos < 0 || pos > size) {
cout << "Invalid position." << endl;
return;
}
if (size == capacity) {
resize(size * 2);
}
for (int i = size - 1; i >= pos; --i) {
data[i + 1] = data[i];
}
data[pos] = value;
++size;
}
// 按位删除元素
void removeAt(int pos) {
if (pos < 0 || pos >= size) {
cout << "Invalid position." << endl;
return;
}
for (int i = pos; i < size - 1; ++i) {
data[i] = data[i + 1];
}
--size;
if (size < capacity / 4 && capacity > 2) {
resize(capacity / 2); // 裁剪内存
}
}
// 按位获取元素
int get(int pos) const {
if (pos < 0 || pos >= size) {
throw out_of_range("Index out of range.");
}
return data[pos];
}
private:
// 扩容操作
void resize(int new_capacity) {
int* newData = new int[new_capacity];
for (int i = 0; i < size; ++i) {
newData[i] = data[i];
}
delete[] data;
data = newData;
capacity = new_capacity;
}
~SeqList() {
if (data != nullptr) {
delete[] data;
data = nullptr;
}
}
};
// 两个顺序表的并操作(不允许重复元素)
void mergeWithoutDuplicates(SeqList& listA, SeqList& listB) {
for (int i = 0; i < listB.size(); ++i) {
if (!listA.search(listB.get(i))) {
listA.insertAt(listA.size(), listB.get(i));
}
}
}
// 两个有序顺序表的合并操作(允许重复元素)
void mergeWithDuplicates(SeqList& orderedListA, SeqList& orderedListB) {
int i = 0, j = 0;
while (i < orderedListA.size() && j < orderedListB.size()) {
if (orderedListA.get(i) <= orderedListB.get(j)) {
orderedListA.insertAt(orderedListA.size(), orderedListA.get(i));
i++;
} else {
orderedListA.insertAt(orderedListA.size(), orderedListB.get(j));
j++;
}
}
while (i < orderedListA.size()) {
orderedListA.insertAt(orderedListA.size(), orderedListA.get(i));
i++;
}
while (j < orderedListB.size()) {
orderedListA.insertAt(orderedListA.size(), orderedListB.get(j));
j++;
}
}
```
阅读全文
相关推荐
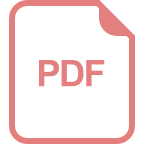
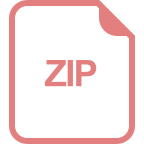
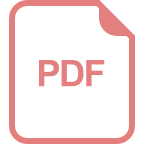
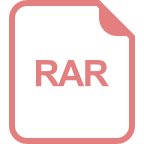
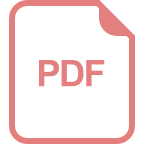
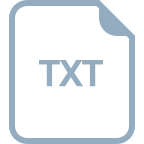
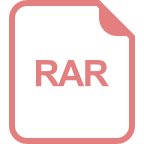
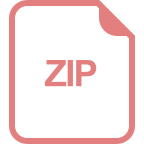
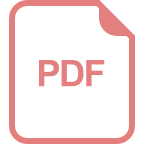
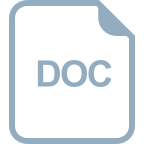
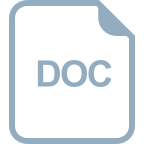
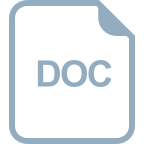
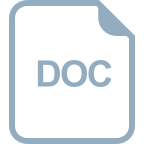
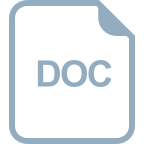
